#include <UserRequest.h>
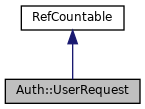
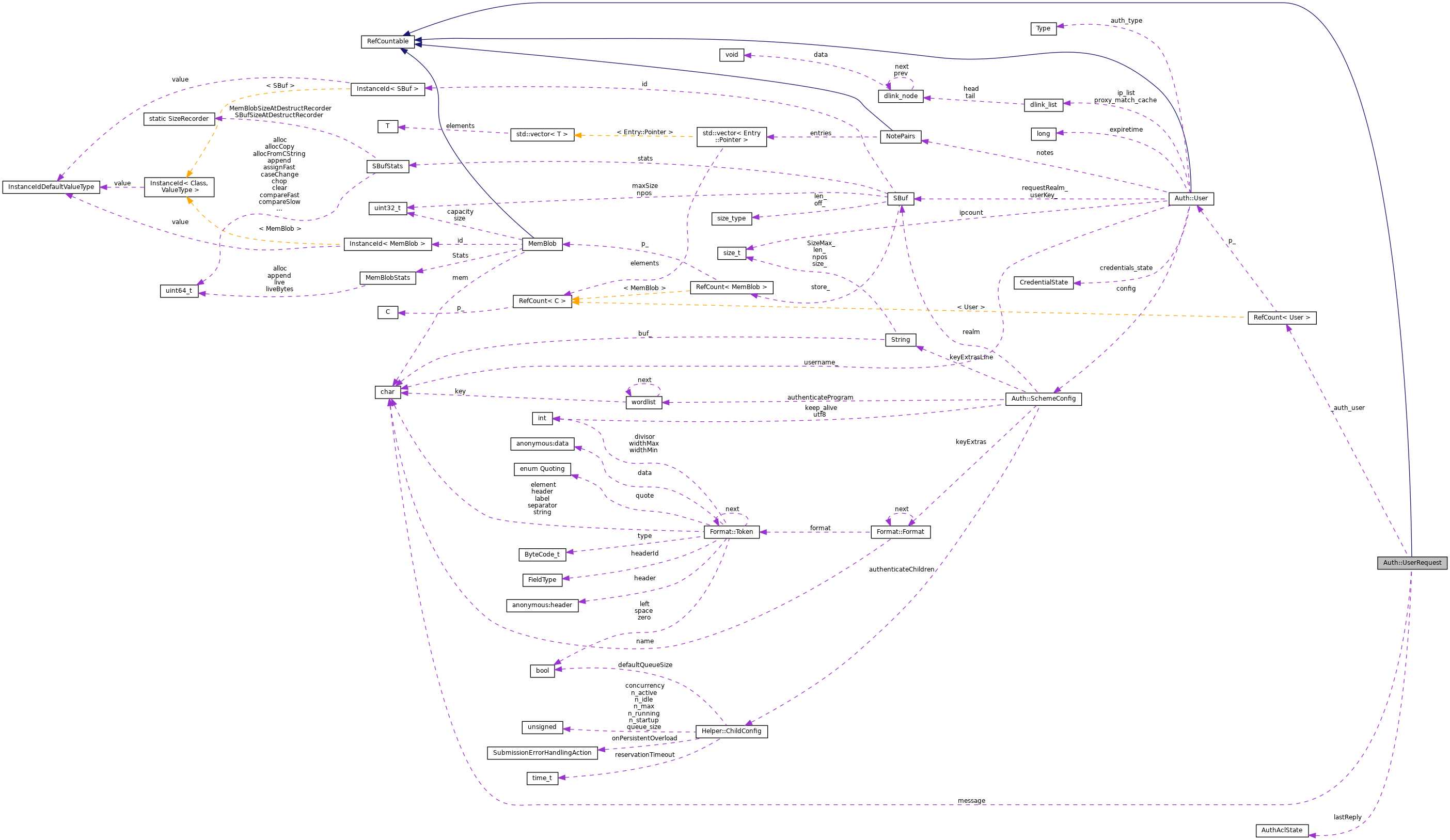
Public Types | |
typedef RefCount< Auth::UserRequest > | Pointer |
Public Member Functions | |
UserRequest () | |
~UserRequest () override | |
void * | operator new (size_t byteCount) |
void | operator delete (void *address) |
Direction | direction () |
virtual int | authenticated () const =0 |
bool | valid () const |
virtual void | authenticate (HttpRequest *request, ConnStateData *conn, Http::HdrType type)=0 |
virtual Direction | module_direction ()=0 |
virtual void | addAuthenticationInfoHeader (HttpReply *rep, int accel) |
virtual void | addAuthenticationInfoTrailer (HttpReply *rep, int accel) |
virtual void | releaseAuthServer () |
virtual User::Pointer | user () |
virtual const User::Pointer | user () const |
virtual void | user (User::Pointer aUser) |
void | start (HttpRequest *request, AccessLogEntry::Pointer &al, AUTHCB *handler, void *data) |
char const * | denyMessage (char const *const default_message=nullptr) const |
void | setDenyMessage (char const *) |
char const * | getDenyMessage () const |
char const * | username () const |
Scheme::Pointer | scheme () const |
virtual const char * | connLastHeader () |
virtual const char * | credentialsStr ()=0 |
const char * | helperRequestKeyExtras (HttpRequest *, AccessLogEntry::Pointer &al) |
void | denyMessageFromHelper (char const *proto, const Helper::Reply &reply) |
Sets the reason of 'authentication denied' helper response. More... | |
Static Public Member Functions | |
static AuthAclState | tryToAuthenticateAndSetAuthUser (UserRequest::Pointer *aUR, Http::HdrType, HttpRequest *, ConnStateData *, Ip::Address &, AccessLogEntry::Pointer &) |
static void | AddReplyAuthHeader (HttpReply *rep, UserRequest::Pointer auth_user_request, HttpRequest *request, int accelerated, int internal) |
Add the appropriate [Proxy-]Authenticate header to the given reply. More... | |
Public Attributes | |
User::Pointer | _auth_user |
Protected Member Functions | |
virtual void | startHelperLookup (HttpRequest *request, AccessLogEntry::Pointer &al, AUTHCB *handler, void *data)=0 |
Static Private Member Functions | |
static AuthAclState | authenticate (UserRequest::Pointer *auth_user_request, Http::HdrType headertype, HttpRequest *request, ConnStateData *conn, Ip::Address &src_addr, AccessLogEntry::Pointer &al) |
Private Attributes | |
char * | message |
AuthAclState | lastReply |
Detailed Description
This is a short lived structure is the visible aspect of the authentication framework.
It and its children hold the state data while processing authentication for a client request. The AuthenticationStateData object is merely a CBDATA wrapper for one of these.
Definition at line 77 of file UserRequest.h.
Member Typedef Documentation
◆ Pointer
Definition at line 80 of file UserRequest.h.
Constructor & Destructor Documentation
◆ UserRequest()
Auth::UserRequest::UserRequest | ( | ) |
Definition at line 92 of file UserRequest.cc.
References debugs.
◆ ~UserRequest()
|
override |
Definition at line 100 of file UserRequest.cc.
Member Function Documentation
◆ addAuthenticationInfoHeader()
Definition at line 197 of file UserRequest.cc.
Referenced by AddReplyAuthHeader().
◆ addAuthenticationInfoTrailer()
Definition at line 201 of file UserRequest.cc.
◆ AddReplyAuthHeader()
|
static |
Definition at line 479 of file UserRequest.cc.
References addAuthenticationInfoHeader(), AUTH_ACL_CANNOT_AUTHENTICATE, AUTH_AUTHENTICATED, Http::BAD_HDR, Auth::User::config, Auth::CRED_CHALLENGE, debugs, direction(), Auth::SchemeConfig::fixHeader(), lastReply, Http::PROXY_AUTHENTICATE, scheme(), schemesConfig(), Http::scProxyAuthenticationRequired, Http::scUnauthorized, HttpReply::sline, Http::StatusLine::status(), user(), and Http::WWW_AUTHENTICATE.
Referenced by clientReplyContext::buildReplyHeader().
◆ authenticate() [1/2]
|
pure virtual |
Referenced by authenticateAuthenticateUser().
◆ authenticate() [2/2]
|
staticprivate |
Definition at line 275 of file UserRequest.cc.
References assert, AUTH_ACL_CHALLENGE, AUTH_ACL_HELPER, AUTH_AUTHENTICATED, HttpRequest::auth_user_request, authenticateAuthenticateUser(), authenticateAuthUserRequestSetIp(), authenticateUserAuthenticated(), authTryGetUser(), conn, Auth::SchemeConfig::CreateAuthUser(), Auth::CRED_CHALLENGE, Auth::CRED_ERROR, Auth::CRED_LOOKUP, Auth::CRED_VALID, DBG_IMPORTANT, debugs, Auth::SchemeConfig::Find(), HttpHeader::getStr(), and Http::Message::header.
◆ authenticated()
|
pure virtual |
Used by squid to determine whether the auth scheme has successfully authenticated the user request.
- Return values
-
true User has successfully been authenticated. false Timeouts on cached credentials have occurred or for any reason the credentials are not valid.
Referenced by authenticateUserAuthenticated().
◆ connLastHeader()
|
virtual |
Definition at line 209 of file UserRequest.cc.
References fatal().
◆ credentialsStr()
|
pure virtual |
The string representation of the credentials send by client
Referenced by Format::Format::assemble().
◆ denyMessage()
char const * Auth::UserRequest::denyMessage | ( | char const *const | default_message = nullptr | ) | const |
Definition at line 127 of file UserRequest.cc.
Referenced by ClientRequestContext::clientAccessCheckDone(), ErrorState::compileLegacyCode(), and ErrorState::Dump().
◆ denyMessageFromHelper()
void Auth::UserRequest::denyMessageFromHelper | ( | char const * | proto, |
const Helper::Reply & | reply | ||
) |
Definition at line 564 of file UserRequest.cc.
References SBuf::append(), SBuf::c_str(), NotePairs::find(), and Helper::Reply::notes.
◆ direction()
Auth::Direction Auth::UserRequest::direction | ( | ) |
Used by squid to determine what the next step in performing authentication for a given scheme is.
- Return values
-
CRED_ERROR ERROR in the auth module. Cannot determine request direction. CRED_LOOKUP The auth module needs to send data to an external helper. Squid will prepare for a callback on the request and call the AUTHSSTART function. CRED_VALID The auth module has all the information it needs to perform the authentication and provide a succeed/fail result. CRED_CHALLENGE The auth module needs to send a new challenge to the request originator. Squid will return the appropriate status code (401 or 407) and call the registered FixError function to allow the auth module to insert it's challenge.
Definition at line 185 of file UserRequest.cc.
References authenticateUserAuthenticated(), Auth::CRED_ERROR, and Auth::CRED_VALID.
Referenced by AddReplyAuthHeader().
◆ getDenyMessage()
char const * Auth::UserRequest::getDenyMessage | ( | ) | const |
Possibly overridable in future
Definition at line 121 of file UserRequest.cc.
◆ helperRequestKeyExtras()
const char * Auth::UserRequest::helperRequestKeyExtras | ( | HttpRequest * | request, |
AccessLogEntry::Pointer & | al | ||
) |
Definition at line 547 of file UserRequest.cc.
References HttpRequest::auth_user_request, MemBuf::content(), debugs, and MemBuf::reset().
◆ module_direction()
|
pure virtual |
◆ operator delete()
void Auth::UserRequest::operator delete | ( | void * | address | ) |
Definition at line 87 of file UserRequest.cc.
References fatal().
◆ operator new()
void * Auth::UserRequest::operator new | ( | size_t | byteCount | ) |
Definition at line 80 of file UserRequest.cc.
References fatal().
◆ releaseAuthServer()
|
virtual |
Definition at line 205 of file UserRequest.cc.
Referenced by ConnStateData::setAuth().
◆ scheme()
Auth::Scheme::Pointer Auth::UserRequest::scheme | ( | ) | const |
Definition at line 541 of file UserRequest.cc.
References Auth::Scheme::Find().
Referenced by AddReplyAuthHeader().
◆ setDenyMessage()
void Auth::UserRequest::setDenyMessage | ( | char const * | aString | ) |
Possibly overridable in future
Definition at line 114 of file UserRequest.cc.
◆ start()
void Auth::UserRequest::start | ( | HttpRequest * | request, |
AccessLogEntry::Pointer & | al, | ||
AUTHCB * | handler, | ||
void * | data | ||
) |
Start an asynchronous helper lookup to verify the user credentials
Uses startHelperLookup() for scheme-specific actions.
The given callback will be called when the auth module has performed it's external activities.
- Parameters
-
handler Handler to process the callback when its run data CBDATA for handler
Definition at line 44 of file UserRequest.cc.
References assert, debugs, and handler().
Referenced by ProxyAuthLookup::checkForAsync().
◆ startHelperLookup()
|
protectedpure virtual |
The scheme-specific actions to be performed when sending helper lookup.
◆ tryToAuthenticateAndSetAuthUser()
|
static |
Locate user credentials in one of several locations. Begin authentication if needed.
Credentials may be found in one of the following locations (listed by order of preference):
- the source passed as parameter aUR
- cached in the HttpRequest parameter from a previous authentication of this request
- cached in the ConnStateData parameter from a previous authentication of this connection (only applies to some situations. ie NTLM, Negotiate, Kerberos auth schemes, or decrypted SSL requests from inside an authenticated CONNECT tunnel)
- cached in the user credentials cache from a previous authentication of the same credentials (only applies to cacheable authentication methods, ie Basic auth)
- new credentials created from HTTP headers in this request
The found credentials are returned in aUR and if successfully authenticated may now be cached in one or more of the above locations.
- Returns
- Some AUTH_ACL_* state
Definition at line 437 of file UserRequest.cc.
References AUTH_ACL_CANNOT_AUTHENTICATE, AUTH_ACL_HELPER, AUTH_AUTHENTICATED, HttpRequest::auth_user_request, authenticate(), authTryGetUser(), conn, and lastReply.
Referenced by AuthenticateAcl().
◆ user() [1/3]
|
inlinevirtual |
Definition at line 143 of file UserRequest.h.
References _auth_user.
Referenced by AddReplyAuthHeader(), authDigestLogUsername(), authenticateAuthUserRequestClearIp(), authenticateAuthUserRequestIPCount(), authenticateAuthUserRequestRemoveIp(), authenticateAuthUserRequestSetIp(), authTryGetUser(), DelayUser::id(), ACLProxyAuth::matchProxyAuth(), and username().
◆ user() [2/3]
|
inlinevirtual |
Definition at line 144 of file UserRequest.h.
References _auth_user.
◆ user() [3/3]
|
inlinevirtual |
Definition at line 145 of file UserRequest.h.
References _auth_user.
◆ username()
char const * Auth::UserRequest::username | ( | ) | const |
Squid does not make assumptions about where the username is stored. This function must return a pointer to a NULL terminated string to be used in logging the request. The string should NOT be allocated each time this function is called.
- Return values
-
NULL No username/usercode is known. * Null-terminated username string.
Definition at line 32 of file UserRequest.cc.
References user().
Referenced by Format::Format::assemble(), ErrorState::compileLegacyCode(), Log::Format::HttpdCombined(), Log::Format::HttpdCommon(), httpFixupAuthentication(), Adaptation::Icap::ModXact::makeUsernameHeader(), ACLMaxUserIP::match(), ACLProxyAuth::matchForCache(), peerUserHashSelectParent(), Log::Format::SquidIcap(), Log::Format::SquidNative(), statClientRequests(), and Adaptation::Ecap::XactionRep::usernameValue().
◆ valid()
bool Auth::UserRequest::valid | ( | ) | const |
Check a auth_user pointer for validity. Does not check passwords, just data sensability. Broken or Unknown auth_types are not valid for use...
- Return values
-
false User credentials are missing. false User credentials use an unknown scheme type. false User credentials are broken for their scheme. true User credentials exist and may be able to authenticate.
Definition at line 53 of file UserRequest.cc.
References Auth::AUTH_BROKEN, Auth::AUTH_UNKNOWN, and debugs.
Referenced by authenticateUserAuthenticated(), ProxyAuthLookup::checkForAsync(), and ProxyAuthLookup::LookupDone().
Member Data Documentation
◆ _auth_user
User::Pointer Auth::UserRequest::_auth_user |
This is the object passed around by client_side and acl functions it has request specific data, and links to user specific data the user
Definition at line 93 of file UserRequest.h.
Referenced by user().
◆ lastReply
|
private |
We only attempt authentication once per http request. This is to allow multiple auth acl references from different _access areas when using connection based authentication
Definition at line 234 of file UserRequest.h.
Referenced by AddReplyAuthHeader(), and tryToAuthenticateAndSetAuthUser().
◆ message
|
private |
return a message on the 407 error pages
Definition at line 227 of file UserRequest.h.
The documentation for this class was generated from the following files:
- src/auth/UserRequest.h
- src/auth/UserRequest.cc