a portable locking-aware exception-friendly file (with RAII API) More...
#include <File.h>
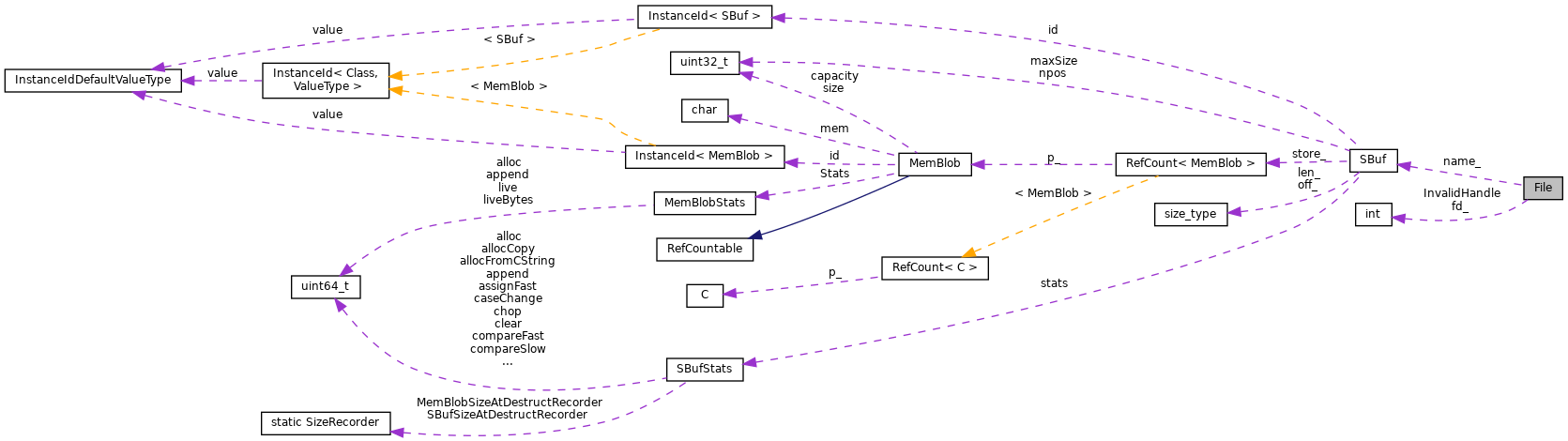
Public Types | |
typedef FileOpeningConfig | Be |
convenient shorthand for File() callers More... | |
Public Member Functions | |
File (const SBuf &aFilename, const FileOpeningConfig &cfg) | |
opens More... | |
~File () | |
closes More... | |
File (const File &)=delete | |
File & | operator= (const File &)=delete |
File (File &&other) | |
File & | operator= (File &&other) |
const SBuf & | name () const |
void | truncate () |
makes the file size (and the current I/O offset) zero More... | |
SBuf | readSmall (SBuf::size_type minBytes, SBuf::size_type maxBytes) |
read(2) for small files More... | |
void | writeAll (const SBuf &data) |
write(2) with a "wrote everything" check More... | |
void | synchronize () |
fsync(2) More... | |
Static Public Member Functions | |
static File * | Optional (const SBuf &aName, const FileOpeningConfig &cfg) |
Protected Member Functions | |
bool | isOpen () const |
void | open (const FileOpeningConfig &cfg) |
opens (or creates) the file More... | |
void | lock (const FileOpeningConfig &cfg) |
calls lockOnce() as many times as necessary (including zero) More... | |
void | lockOnce (const FileOpeningConfig &cfg) |
locks, blocking or returning immediately depending on the lock waiting mode More... | |
void | close () |
SBuf | sysCallFailure (const char *callName, const SBuf &error) const |
SBuf | sysCallError (const char *callName, const int savedErrno) const |
Private Types | |
typedef int | Handle |
Private Attributes | |
SBuf | name_ |
location on disk More... | |
Handle | fd_ = InvalidHandle |
OS-specific file handle. More... | |
Static Private Attributes | |
static const Handle | InvalidHandle = -1 |
Detailed Description
Member Typedef Documentation
◆ Be
typedef FileOpeningConfig File::Be |
◆ Handle
|
private |
Constructor & Destructor Documentation
◆ File() [1/3]
File::File | ( | const SBuf & | aFilename, |
const FileOpeningConfig & | cfg | ||
) |
◆ ~File()
◆ File() [2/3]
|
delete |
◆ File() [3/3]
Member Function Documentation
◆ close()
|
protected |
Definition at line 196 of file File.cc.
References close(), DBG_IMPORTANT, debugs, fd_, isOpen(), sysCallError(), and sysCallFailure().
◆ isOpen()
|
inlineprotected |
◆ lock()
|
protected |
Definition at line 321 of file File.cc.
References debugs, FileOpeningConfig::lockAttempts, lockOnce(), Must, and FileOpeningConfig::retryGapUsec.
Referenced by File().
◆ lockOnce()
|
protected |
Definition at line 342 of file File.cc.
References debugs, fd_, FileOpeningConfig::flockMode, name_, sysCallError(), sysCallFailure(), and TexcHere.
Referenced by lock().
◆ name()
◆ open()
|
protected |
Definition at line 171 of file File.cc.
References SBuf::c_str(), FileOpeningConfig::creationMask, enter_suid(), fd_, InvalidHandle, leave_suid(), name_, open(), FileOpeningConfig::openFlags, FileOpeningConfig::openMode, sysCallError(), sysCallFailure(), and TexcHere.
◆ operator=() [1/2]
◆ operator=() [2/2]
◆ Optional()
|
static |
◆ readSmall()
SBuf File::readSmall | ( | SBuf::size_type | minBytes, |
SBuf::size_type | maxBytes | ||
) |
Definition at line 241 of file File.cc.
References assert, fd_, SBuf::length(), Must, SBuf::rawAppendFinish(), SBuf::rawAppendStart(), sysCallError(), sysCallFailure(), and TexcHere.
Referenced by GetOtherPid().
◆ synchronize()
void File::synchronize | ( | ) |
Definition at line 304 of file File.cc.
References fd_, sysCallError(), sysCallFailure(), and TexcHere.
Referenced by Instance::WriteOurPid().
◆ sysCallError()
- Returns
- a description of an errno-based system call failure
Definition at line 372 of file File.cc.
References sysCallFailure(), and xstrerr().
Referenced by close(), lockOnce(), open(), readSmall(), synchronize(), truncate(), and writeAll().
◆ sysCallFailure()
- Returns
- a description a system call-related failure
Definition at line 365 of file File.cc.
References error(), name_, and ToSBuf().
Referenced by close(), lockOnce(), open(), readSmall(), synchronize(), sysCallError(), truncate(), and writeAll().
◆ truncate()
void File::truncate | ( | ) |
Definition at line 215 of file File.cc.
References fd_, sysCallError(), sysCallFailure(), and TexcHere.
Referenced by Instance::WriteOurPid().
◆ writeAll()
void File::writeAll | ( | const SBuf & | data | ) |
Definition at line 280 of file File.cc.
References fd_, SBuf::length(), SBuf::rawContent(), sysCallError(), sysCallFailure(), and TexcHere.
Referenced by Instance::WriteOurPid().
Member Data Documentation
◆ fd_
|
private |
Definition at line 123 of file File.h.
Referenced by close(), isOpen(), lockOnce(), open(), operator=(), readSmall(), synchronize(), truncate(), and writeAll().
◆ InvalidHandle
|
staticprivate |
◆ name_
|
private |
Definition at line 113 of file File.h.
Referenced by File(), ~File(), lockOnce(), name(), open(), and sysCallFailure().
The documentation for this class was generated from the following files: