#include <QosConfig.h>
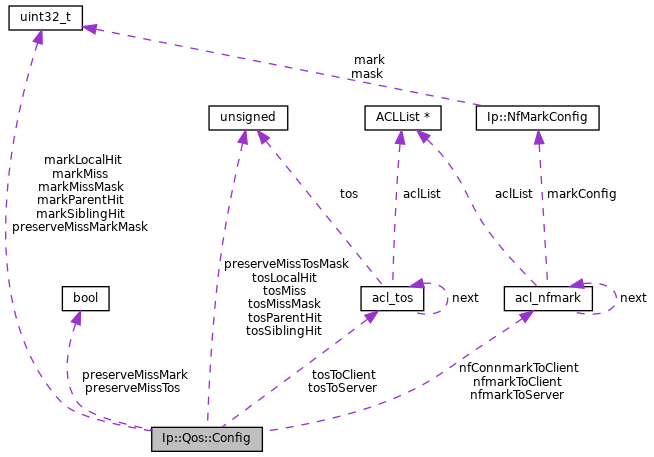
Public Member Functions | |
Config () | |
~Config () | |
void | parseConfigLine () |
void | dumpConfigLine (char *entry, const char *name) const |
bool | isHitTosActive () const |
Whether we should modify TOS flags based on cache hits and misses. More... | |
bool | isHitNfmarkActive () const |
Whether we should modify netfilter marks based on cache hits and misses. More... | |
bool | isAclNfmarkActive () const |
bool | isAclTosActive () const |
Public Attributes | |
tos_t | tosLocalHit |
TOS value to apply to local cache hits. More... | |
tos_t | tosSiblingHit |
TOS value to apply to hits from siblings. More... | |
tos_t | tosParentHit |
TOS value to apply to hits from parent. More... | |
tos_t | tosMiss |
TOS value to apply to cache misses. More... | |
tos_t | tosMissMask |
Mask for TOS value to apply to cache misses. Applied to the tosMiss value. More... | |
bool | preserveMissTos |
Whether to preserve the TOS value of the inbound packet for misses. More... | |
tos_t | preserveMissTosMask |
The mask to apply when preserving the TOS of misses. Applies to preserved value from upstream. More... | |
nfmark_t | markLocalHit |
Netfilter mark value to apply to local cache hits. More... | |
nfmark_t | markSiblingHit |
Netfilter mark value to apply to hits from siblings. More... | |
nfmark_t | markParentHit |
Netfilter mark value to apply to hits from parent. More... | |
nfmark_t | markMiss |
Netfilter mark value to apply to cache misses. More... | |
nfmark_t | markMissMask |
Mask for netfilter mark value to apply to cache misses. Applied to the markMiss value. More... | |
bool | preserveMissMark |
Whether to preserve netfilter mark value of inbound connection. More... | |
nfmark_t | preserveMissMarkMask |
The mask to apply when preserving the netfilter mark of misses. Applied to preserved value from upstream. More... | |
acl_tos * | tosToServer |
The TOS that packets to the web server should be marked with, based on ACL. More... | |
acl_tos * | tosToClient |
The TOS that packets to the client should be marked with, based on ACL. More... | |
acl_nfmark * | nfmarkToServer |
The MARK that packets to the web server should be marked with, based on ACL. More... | |
acl_nfmark * | nfmarkToClient |
The MARK that packets to the client should be marked with, based on ACL. More... | |
acl_nfmark * | nfConnmarkToClient = nullptr |
The CONNMARK that the client connection should be marked with, based on ACL. More... | |
Detailed Description
QOS configuration class. Contains all the parameters for QOS functions as well as functions to check whether either TOS or MARK QOS is enabled.
Definition at line 174 of file QosConfig.h.
Constructor & Destructor Documentation
◆ Config()
Ip::Qos::Config::Config | ( | ) |
Definition at line 285 of file QosConfig.cc.
◆ ~Config()
|
inline |
Definition at line 179 of file QosConfig.h.
Member Function Documentation
◆ dumpConfigLine()
void Ip::Qos::Config::dumpConfigLine | ( | char * | entry, |
const char * | name | ||
) | const |
Dump all the configuration values
NOTE: Due to the low-level nature of the library these objects are part of the dump function must be self-contained. which means no StoreEntry references. Just a basic char* buffer.
NOTE: Due to the low-level nature of the library these objects are part of the dump function must be self-contained. which means no StoreEntry references. Just a basic char* buffer.
Definition at line 468 of file QosConfig.cc.
◆ isAclNfmarkActive()
bool Ip::Qos::Config::isAclNfmarkActive | ( | ) | const |
Iterates through any outgoing_nfmark or clientside_nfmark configuration parameters to find out if any Netfilter marking is required. This function is used on initialisation to define capabilities required (Netfilter marking requires CAP_NET_ADMIN).
Definition at line 610 of file QosConfig.cc.
References Ip::NfMarkConfig::isEmpty(), acl_nfmark::markConfig, and acl_nfmark::next.
◆ isAclTosActive()
bool Ip::Qos::Config::isAclTosActive | ( | ) | const |
Iterates through any outgoing_tos or clientside_tos configuration parameters to find out if packets should be marked with TOS flags.
Definition at line 627 of file QosConfig.cc.
References acl_tos::next, and acl_tos::tos.
◆ isHitNfmarkActive()
|
inline |
Definition at line 198 of file QosConfig.h.
References markLocalHit, markMiss, markParentHit, markSiblingHit, and preserveMissMark.
◆ isHitTosActive()
|
inline |
Definition at line 193 of file QosConfig.h.
References preserveMissTos, tosLocalHit, tosMiss, tosParentHit, and tosSiblingHit.
◆ parseConfigLine()
void Ip::Qos::Config::parseConfigLine | ( | ) |
Definition at line 296 of file QosConfig.cc.
References DBG_CRITICAL, DBG_IMPORTANT, debugs, max(), ConfigParser::NextToken(), self_destruct(), and xstrtoui().
Member Data Documentation
◆ markLocalHit
nfmark_t Ip::Qos::Config::markLocalHit |
Definition at line 224 of file QosConfig.h.
Referenced by isHitNfmarkActive().
◆ markMiss
nfmark_t Ip::Qos::Config::markMiss |
Definition at line 227 of file QosConfig.h.
Referenced by Ip::Qos::doNfmarkLocalMiss(), and isHitNfmarkActive().
◆ markMissMask
nfmark_t Ip::Qos::Config::markMissMask |
Definition at line 228 of file QosConfig.h.
Referenced by Ip::Qos::doNfmarkLocalMiss().
◆ markParentHit
nfmark_t Ip::Qos::Config::markParentHit |
Definition at line 226 of file QosConfig.h.
Referenced by Ip::Qos::doNfmarkLocalMiss(), and isHitNfmarkActive().
◆ markSiblingHit
nfmark_t Ip::Qos::Config::markSiblingHit |
Definition at line 225 of file QosConfig.h.
Referenced by Ip::Qos::doNfmarkLocalMiss(), and isHitNfmarkActive().
◆ nfConnmarkToClient
acl_nfmark* Ip::Qos::Config::nfConnmarkToClient = nullptr |
Definition at line 236 of file QosConfig.h.
◆ nfmarkToClient
acl_nfmark* Ip::Qos::Config::nfmarkToClient |
Definition at line 235 of file QosConfig.h.
◆ nfmarkToServer
acl_nfmark* Ip::Qos::Config::nfmarkToServer |
Definition at line 234 of file QosConfig.h.
◆ preserveMissMark
bool Ip::Qos::Config::preserveMissMark |
Definition at line 229 of file QosConfig.h.
Referenced by isHitNfmarkActive().
◆ preserveMissMarkMask
nfmark_t Ip::Qos::Config::preserveMissMarkMask |
Definition at line 230 of file QosConfig.h.
Referenced by Ip::Qos::doNfmarkLocalMiss().
◆ preserveMissTos
bool Ip::Qos::Config::preserveMissTos |
Definition at line 221 of file QosConfig.h.
Referenced by isHitTosActive().
◆ preserveMissTosMask
tos_t Ip::Qos::Config::preserveMissTosMask |
Definition at line 222 of file QosConfig.h.
Referenced by Ip::Qos::doTosLocalMiss().
◆ tosLocalHit
tos_t Ip::Qos::Config::tosLocalHit |
Definition at line 216 of file QosConfig.h.
Referenced by isHitTosActive().
◆ tosMiss
tos_t Ip::Qos::Config::tosMiss |
Definition at line 219 of file QosConfig.h.
Referenced by Ip::Qos::doTosLocalMiss(), and isHitTosActive().
◆ tosMissMask
tos_t Ip::Qos::Config::tosMissMask |
Definition at line 220 of file QosConfig.h.
Referenced by Ip::Qos::doTosLocalMiss().
◆ tosParentHit
tos_t Ip::Qos::Config::tosParentHit |
Definition at line 218 of file QosConfig.h.
Referenced by Ip::Qos::doTosLocalMiss(), and isHitTosActive().
◆ tosSiblingHit
tos_t Ip::Qos::Config::tosSiblingHit |
Definition at line 217 of file QosConfig.h.
Referenced by Ip::Qos::doTosLocalMiss(), and isHitTosActive().
◆ tosToClient
acl_tos* Ip::Qos::Config::tosToClient |
Definition at line 233 of file QosConfig.h.
◆ tosToServer
acl_tos* Ip::Qos::Config::tosToServer |
Definition at line 232 of file QosConfig.h.
The documentation for this class was generated from the following files:
- src/ip/QosConfig.h
- src/ip/QosConfig.cc