#include <PageStack.h>
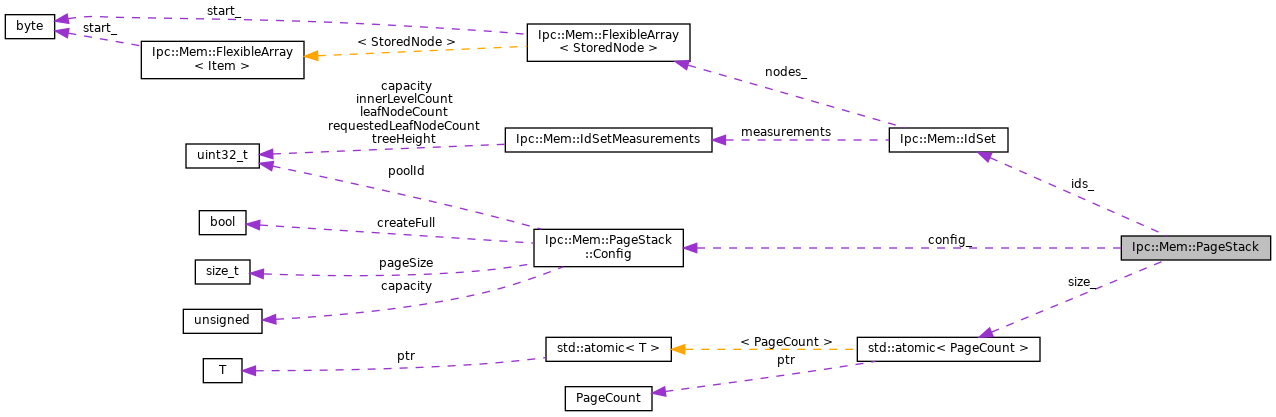
Classes | |
class | Config |
PageStack construction and SharedMemorySize calculation parameters. More... | |
Public Types | |
typedef std::atomic< size_t > | Levels_t |
typedef unsigned int | PageCount |
the number of (free and/or used) pages in a stack More... | |
Public Member Functions | |
PageStack (const Config &) | |
PageCount | capacity () const |
size_t | pageSize () const |
PageCount | size () const |
an approximate number of free pages More... | |
bool | pop (PageId &page) |
sets value and returns true unless no free page numbers are found More... | |
void | push (PageId &page) |
makes value available as a free page number to future pop() callers More... | |
bool | pageIdIsValid (const PageId &page) const |
size_t | sharedMemorySize () const |
size_t | stackSize () const |
size_t | levelsPaddingSize () const |
Static Public Member Functions | |
static size_t | SharedMemorySize (const Config &) |
total shared memory size required to share More... | |
static size_t | StackSize (const PageCount capacity) |
static size_t | LevelsPaddingSize (const PageCount capacity) |
static PoolId | IdForMemStoreSpace () |
stack of free cache_mem slot positions More... | |
static PoolId | IdForMultipurposePool () |
multipurpose PagePool of shared memory pages More... | |
static PoolId | IdForSwapDirSpace (const int dirIdx) |
stack of free rock cache_dir slot numbers More... | |
Private Attributes | |
const Config | config_ |
std::atomic< PageCount > | size_ |
a lower bound for the number of free pages (for debugging purposes) More... | |
IdSet | ids_ |
free pages (valid with positive capacity_) More... | |
Detailed Description
Atomic container of "free" PageIds. Detects (some) double-free bugs. Assumptions: All page numbers are unique, positive, with a known maximum. A pushed page may not become available immediately but is never truly lost.
Definition at line 108 of file PageStack.h.
Member Typedef Documentation
◆ Levels_t
typedef std::atomic<size_t> Ipc::Mem::PageStack::Levels_t |
Definition at line 111 of file PageStack.h.
◆ PageCount
typedef unsigned int Ipc::Mem::PageStack::PageCount |
Definition at line 117 of file PageStack.h.
Constructor & Destructor Documentation
◆ PageStack()
|
explicit |
Definition at line 430 of file PageStack.cc.
References Ipc::Mem::PageStack::Config::capacity, config_, Ipc::Mem::PageStack::Config::createFull, ids_, Ipc::Mem::IdSet::makeFullBeforeSharing(), and size_.
Member Function Documentation
◆ capacity()
|
inline |
Definition at line 135 of file PageStack.h.
References Ipc::Mem::PageStack::Config::capacity, and config_.
◆ IdForMemStoreSpace()
|
inlinestatic |
The following functions return PageStack IDs for the corresponding PagePool or a similar PageStack user. The exact values are unimportant, but their uniqueness and stability eases debugging.
Definition at line 167 of file PageStack.h.
Referenced by MemStoreRr::create(), and MemStore::noteFreeMapSlice().
◆ IdForMultipurposePool()
|
inlinestatic |
Definition at line 169 of file PageStack.h.
Referenced by SharedMemPagesRr::create().
◆ IdForSwapDirSpace()
Definition at line 171 of file PageStack.h.
Referenced by Rock::SwapDirRr::create(), Rock::Rebuild::freeSlot(), and Rock::SwapDir::noteFreeMapSlice().
◆ levelsPaddingSize()
|
inline |
Definition at line 158 of file PageStack.h.
References Ipc::Mem::PageStack::Config::capacity, config_, and LevelsPaddingSize().
◆ LevelsPaddingSize()
- Returns
- the number of padding bytes to align PagePool::theLevels array
Definition at line 519 of file PageStack.cc.
Referenced by levelsPaddingSize().
◆ pageIdIsValid()
bool Ipc::Mem::PageStack::pageIdIsValid | ( | const PageId & | page | ) | const |
Definition at line 483 of file PageStack.cc.
References Ipc::Mem::PageId::number, and Ipc::Mem::PageId::pool.
◆ pageSize()
|
inline |
Definition at line 136 of file PageStack.h.
References config_, and Ipc::Mem::PageStack::Config::pageSize.
◆ pop()
bool Ipc::Mem::PageStack::pop | ( | PageId & | page | ) |
Definition at line 442 of file PageStack.cc.
References assert, debugs, Ipc::Mem::PageId::number, and Ipc::Mem::PageId::pool.
Referenced by MemStore::reserveSapForWriting().
◆ push()
void Ipc::Mem::PageStack::push | ( | PageId & | page | ) |
Definition at line 465 of file PageStack.cc.
References assert, debugs, and Ipc::Mem::PageId::number.
Referenced by MemStore::noteFreeMapSlice(), and MemStore::reserveSapForWriting().
◆ sharedMemorySize()
size_t Ipc::Mem::PageStack::sharedMemorySize | ( | ) | const |
Definition at line 490 of file PageStack.cc.
◆ SharedMemorySize()
Definition at line 496 of file PageStack.cc.
References Ipc::Mem::PageStack::Config::capacity, Ipc::Mem::PageId::maxPurpose, and Ipc::Mem::PageStack::Config::pageSize.
◆ size()
|
inline |
Definition at line 138 of file PageStack.h.
References size_.
◆ stackSize()
size_t Ipc::Mem::PageStack::stackSize | ( | ) | const |
Definition at line 513 of file PageStack.cc.
◆ StackSize()
shared memory size required only by PageStack, excluding shared counters and page data
Definition at line 504 of file PageStack.cc.
References Ipc::Mem::IdSet::MemorySize().
Member Data Documentation
◆ config_
|
private |
Definition at line 174 of file PageStack.h.
Referenced by PageStack(), capacity(), levelsPaddingSize(), and pageSize().
◆ ids_
|
private |
Definition at line 178 of file PageStack.h.
Referenced by PageStack().
◆ size_
|
private |
Definition at line 176 of file PageStack.h.
Referenced by PageStack(), and size().
The documentation for this class was generated from the following files:
- src/ipc/mem/PageStack.h
- src/ipc/mem/PageStack.cc