#include <Kid.h>
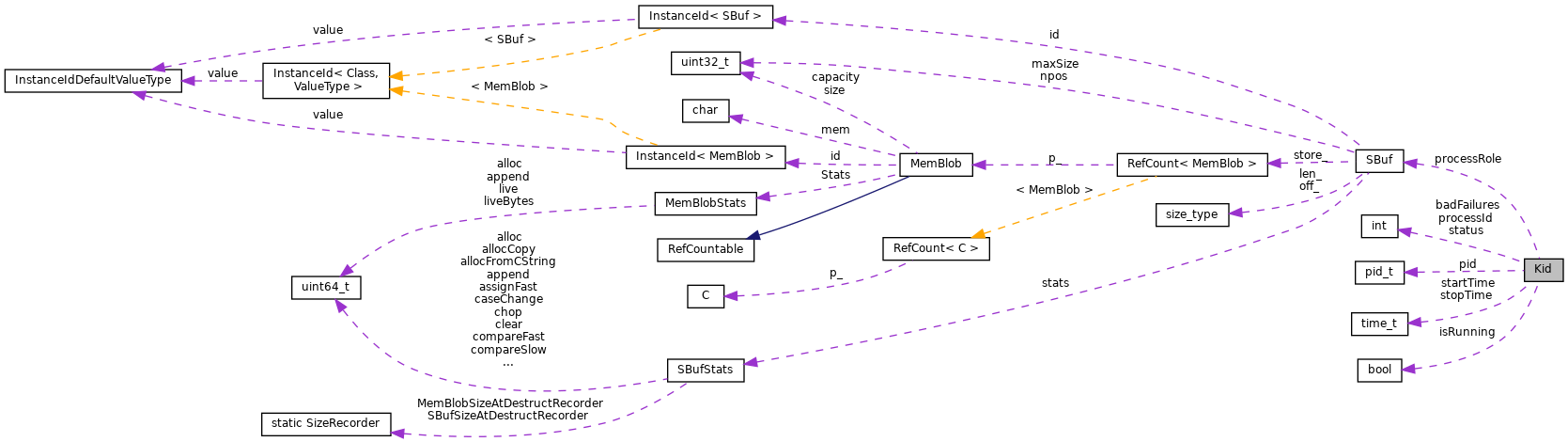
Public Types | |
enum | { badFailureLimit = 4 } |
keep restarting until the number of bad failures exceed this limit More... | |
enum | { fastFailureTimeLimit = 10 } |
slower start failures are not "frequent enough" to be counted as "bad" More... | |
Public Member Functions | |
Kid () | |
Kid (const char *role, const int id) | |
void | start (pid_t cpid) |
called when this kid got started, records PID More... | |
void | stop (PidStatus const exitStatus) |
called when kid terminates, sets exiting status More... | |
bool | running () const |
returns true if tracking of kid is stopped More... | |
bool | shouldRestart () const |
returns true if master should restart this kid More... | |
pid_t | getPid () const |
returns current pid for a running kid and last pid for a stopped kid More... | |
bool | hopeless () const |
whether the failures are "repeated and frequent" More... | |
void | forgetFailures () |
forgets all past failures, ensuring that we are not hopeless() More... | |
time_t | deathDuration () const |
bool | calledExit () const |
returns true if the process terminated normally More... | |
int | exitStatus () const |
returns the exit status of the process More... | |
bool | calledExit (int code) const |
whether the process exited with a given exit status code More... | |
bool | exitedHappy () const |
whether the process exited with code 0 More... | |
bool | signaled () const |
returns true if the kid was terminated by a signal More... | |
int | termSignal () const |
returns the number of the signal that caused the kid to terminate More... | |
bool | signaled (int sgnl) const |
whether the process was terminated by a given signal More... | |
SBuf | processName () const |
returns kid name More... | |
SBuf | gist () const |
Private Member Functions | |
void | reportStopped () const |
describes a recently stopped kid More... | |
Private Attributes | |
SBuf | processRole |
int | processId = 0 |
int | badFailures = 0 |
number of "repeated frequent" failures More... | |
pid_t | pid = -1 |
current (for a running kid) or last (for stopped kid) PID More... | |
time_t | startTime = 0 |
last start time More... | |
time_t | stopTime = 0 |
last termination time More... | |
bool | isRunning = false |
whether the kid is assumed to be alive More... | |
PidStatus | status = 0 |
exit status of a stopped kid More... | |
Detailed Description
Squid child, including current forked process info and info persistent across restarts
Member Enumeration Documentation
◆ anonymous enum
◆ anonymous enum
Constructor & Destructor Documentation
◆ Kid() [1/2]
◆ Kid() [2/2]
Member Function Documentation
◆ calledExit() [1/2]
bool Kid::calledExit | ( | ) | const |
Definition at line 121 of file Kid.cc.
References pid, running(), and status.
Referenced by calledExit(), exitedHappy(), and reportStopped().
◆ calledExit() [2/2]
bool Kid::calledExit | ( | int | code | ) | const |
Definition at line 133 of file Kid.cc.
References calledExit(), code, and exitStatus().
◆ deathDuration()
time_t Kid::deathDuration | ( | ) | const |
- Returns
- the time since process termination
Definition at line 179 of file Kid.cc.
References squid_curtime, and stopTime.
◆ exitedHappy()
bool Kid::exitedHappy | ( | ) | const |
◆ exitStatus()
int Kid::exitStatus | ( | ) | const |
Definition at line 127 of file Kid.cc.
References status.
Referenced by calledExit(), and reportStopped().
◆ forgetFailures()
|
inline |
Definition at line 51 of file Kid.h.
References badFailures.
◆ getPid()
◆ gist()
SBuf Kid::gist | ( | ) | const |
- Returns
- kid's role and ID summary; usable as a –kid parameter value
Definition at line 171 of file Kid.cc.
References SBuf::appendf(), processId, and processRole.
Referenced by processName(), reportStopped(), and watch_child().
◆ hopeless()
bool Kid::hopeless | ( | ) | const |
Definition at line 115 of file Kid.cc.
References badFailureLimit, and badFailures.
Referenced by reportStopped(), and shouldRestart().
◆ processName()
SBuf Kid::processName | ( | ) | const |
- Returns
- kid's role and ID formatted for use as a process name
Definition at line 163 of file Kid.cc.
References SBuf::append(), and gist().
Referenced by watch_child().
◆ reportStopped()
|
private |
Definition at line 66 of file Kid.cc.
References calledExit(), Config, exitStatus(), gist(), hopeless(), SquidConfig::hopelessKidRevivalDelay, pid, signaled(), and termSignal().
Referenced by stop().
◆ running()
bool Kid::running | ( | ) | const |
Definition at line 91 of file Kid.cc.
References isRunning.
Referenced by calledExit(), shouldRestart(), signaled(), start(), and stop().
◆ shouldRestart()
bool Kid::shouldRestart | ( | ) | const |
returns true if master process should restart this kid
Definition at line 97 of file Kid.cc.
References exitedHappy(), hopeless(), running(), shutting_down, and signaled().
Referenced by watch_child().
◆ signaled() [1/2]
bool Kid::signaled | ( | ) | const |
Definition at line 145 of file Kid.cc.
References pid, running(), and status.
Referenced by reportStopped(), shouldRestart(), and signaled().
◆ signaled() [2/2]
bool Kid::signaled | ( | int | sgnl | ) | const |
Definition at line 157 of file Kid.cc.
References signaled(), and termSignal().
◆ start()
void Kid::start | ( | pid_t | cpid | ) |
Definition at line 34 of file Kid.cc.
References assert, isRunning, pid, running(), squid_curtime, startTime, and stopTime.
Referenced by watch_child().
◆ stop()
void Kid::stop | ( | PidStatus const | exitStatus | ) |
Definition at line 47 of file Kid.cc.
References assert, badFailures, fastFailureTimeLimit, isRunning, reportStopped(), running(), squid_curtime, startTime, status, and stopTime.
◆ termSignal()
int Kid::termSignal | ( | ) | const |
Definition at line 151 of file Kid.cc.
References status.
Referenced by reportStopped(), and signaled().
Member Data Documentation
◆ badFailures
|
private |
Definition at line 89 of file Kid.h.
Referenced by forgetFailures(), hopeless(), and stop().
◆ isRunning
|
private |
◆ pid
|
private |
Definition at line 92 of file Kid.h.
Referenced by calledExit(), getPid(), reportStopped(), signaled(), and start().
◆ processId
◆ processRole
◆ startTime
|
private |
◆ status
|
private |
Definition at line 96 of file Kid.h.
Referenced by calledExit(), exitStatus(), signaled(), stop(), and termSignal().
◆ stopTime
|
private |
Definition at line 94 of file Kid.h.
Referenced by deathDuration(), start(), and stop().
The documentation for this class was generated from the following files: