#include <BinaryTokenizer.h>
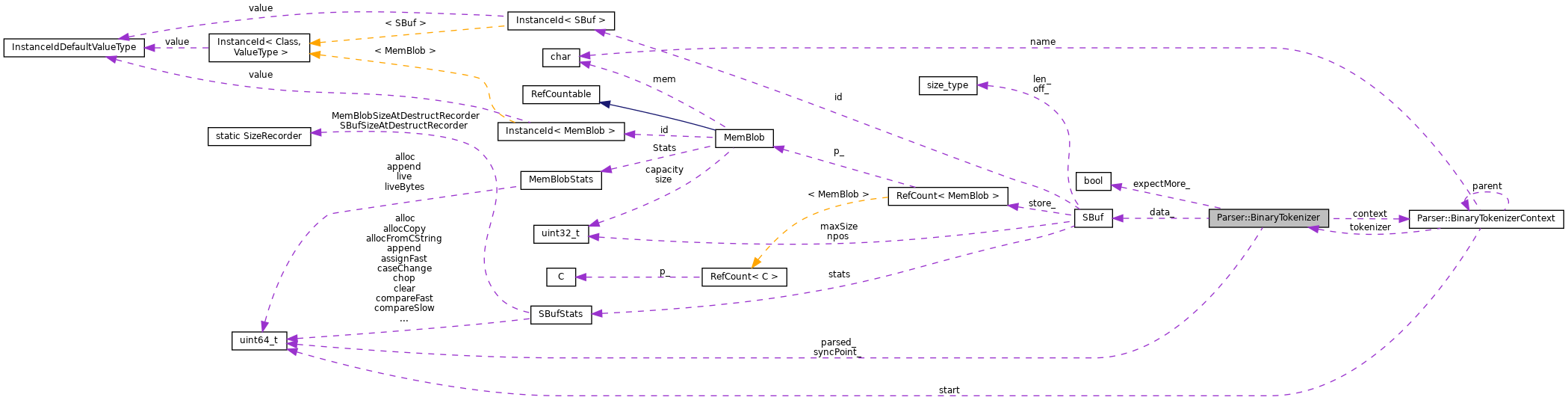
Public Types | |
typedef ::Parser::InsufficientInput | InsufficientInput |
typedef uint64_t | size_type |
Public Member Functions | |
BinaryTokenizer () | |
BinaryTokenizer (const SBuf &data, const bool expectMore=false) | |
void | reset (const SBuf &data, const bool expectMore) |
void | reinput (const SBuf &data, const bool expectMore) |
void | commit () |
make progress: future parsing failures will not rollback beyond this point More... | |
void | rollback () |
resume [incremental] parsing from the last commit point More... | |
bool | atEnd () const |
no more bytes to parse or skip More... | |
uint8_t | uint8 (const char *description) |
parse a single-byte unsigned integer More... | |
uint16_t | uint16 (const char *description) |
parse a two-byte unsigned integer More... | |
uint32_t | uint24 (const char *description) |
parse a three-byte unsigned integer (returned as uint32_t) More... | |
uint32_t | uint32 (const char *description) |
parse a four-byte unsigned integer More... | |
SBuf | area (uint64_t size, const char *description) |
parse size consecutive bytes as an opaque blob More... | |
Ip::Address | inet4 (const char *description) |
interpret the next 4 bytes as a raw in_addr structure More... | |
Ip::Address | inet6 (const char *description) |
interpret the next 16 bytes as a raw in6_addr structure More... | |
SBuf | pstring8 (const char *description) |
up to 255 byte-long p-string More... | |
SBuf | pstring16 (const char *description) |
up to 64 KiB-long p-string More... | |
SBuf | pstring24 (const char *description) |
up to 16 MiB-long p-string! More... | |
void | skip (uint64_t size, const char *description) |
ignore the next size bytes More... | |
uint64_t | parsed () const |
the number of already parsed bytes More... | |
SBuf | leftovers () const |
yet unparsed bytes More... | |
void | got (uint64_t size, const char *description) const |
debugging helper for parsed multi-field structures More... | |
Public Attributes | |
const BinaryTokenizerContext * | context |
debugging: thing being parsed More... | |
Protected Member Functions | |
uint32_t | octet () |
void | want (uint64_t size, const char *description) const |
logs and throws if fewer than size octets remain; no other side effects More... | |
void | got (uint32_t value, uint64_t size, const char *description) const |
debugging helper for parsed number fields More... | |
void | got (const SBuf &value, uint64_t size, const char *description) const |
debugging helper for parsed areas/blobs More... | |
void | got (const Ip::Address &value, uint64_t size, const char *description) const |
debugging helper for parsed addresses More... | |
void | skipped (uint64_t size, const char *description) const |
debugging helper for skipped fields More... | |
Private Member Functions | |
template<class InAddr > | |
Ip::Address | inetAny (const char *description) |
Private Attributes | |
SBuf | data_ |
uint64_t | parsed_ |
number of data bytes parsed or skipped More... | |
uint64_t | syncPoint_ |
where to re-start the next parsing attempt More... | |
bool | expectMore_ |
whether more data bytes may arrive in the future More... | |
Detailed Description
Safely extracts byte-oriented (i.e., non-textual) fields from raw input. Assume that the integers are stored in network byte order. Supports commit points for atomic incremental parsing of multi-part fields. Throws InsufficientInput when more input is needed to parse the next field. Throws on errors.
Definition at line 46 of file BinaryTokenizer.h.
Member Typedef Documentation
◆ InsufficientInput
Definition at line 49 of file BinaryTokenizer.h.
◆ size_type
typedef uint64_t Parser::BinaryTokenizer::size_type |
Definition at line 50 of file BinaryTokenizer.h.
Constructor & Destructor Documentation
◆ BinaryTokenizer() [1/2]
Parser::BinaryTokenizer::BinaryTokenizer | ( | ) |
Definition at line 16 of file BinaryTokenizer.cc.
◆ BinaryTokenizer() [2/2]
|
explicit |
Definition at line 20 of file BinaryTokenizer.cc.
Member Function Documentation
◆ area()
SBuf Parser::BinaryTokenizer::area | ( | uint64_t | size, |
const char * | description | ||
) |
Definition at line 168 of file BinaryTokenizer.cc.
References size, and SBuf::substr().
Referenced by Security::Sslv2Record::Sslv2Record(), Security::HandshakeParser::parseClientHelloHandshakeMessage(), and Security::HandshakeParser::parseVersion2HandshakeMessage().
◆ atEnd()
bool Parser::BinaryTokenizer::atEnd | ( | ) | const |
Definition at line 126 of file BinaryTokenizer.cc.
Referenced by Security::HandshakeParser::parseCiphers(), Security::HandshakeParser::parseClientHelloHandshakeMessage(), Security::HandshakeParser::parseCompressionMethods(), Security::HandshakeParser::parseExtensions(), Security::HandshakeParser::parseServerHelloHandshakeMessage(), Security::HandshakeParser::parseSniExtension(), Security::HandshakeParser::parseSupportedVersionsExtension(), and Security::HandshakeParser::parseV23Ciphers().
◆ commit()
void Parser::BinaryTokenizer::commit | ( | ) |
Definition at line 120 of file BinaryTokenizer.cc.
◆ got() [1/4]
|
protected |
Definition at line 82 of file BinaryTokenizer.cc.
References BinaryTokenizer_tail, debugs, and size.
◆ got() [2/4]
|
protected |
Definition at line 72 of file BinaryTokenizer.cc.
References BinaryTokenizer_tail, debugs, Raw::hex(), SBuf::length(), SBuf::rawContent(), and size.
◆ got() [3/4]
|
protected |
Definition at line 64 of file BinaryTokenizer.cc.
References BinaryTokenizer_tail, debugs, and size.
◆ got() [4/4]
void Parser::BinaryTokenizer::got | ( | uint64_t | size, |
const char * | description | ||
) | const |
Definition at line 56 of file BinaryTokenizer.cc.
References BinaryTokenizer_tail, debugs, and size.
Referenced by Parser::BinaryTokenizerContext::success().
◆ inet4()
Ip::Address Parser::BinaryTokenizer::inet4 | ( | const char * | description | ) |
Definition at line 192 of file BinaryTokenizer.cc.
◆ inet6()
Ip::Address Parser::BinaryTokenizer::inet6 | ( | const char * | description | ) |
Definition at line 198 of file BinaryTokenizer.cc.
◆ inetAny()
|
private |
Definition at line 179 of file BinaryTokenizer.cc.
References size.
◆ leftovers()
|
inline |
Definition at line 108 of file BinaryTokenizer.h.
References data_, parsed_, and SBuf::substr().
◆ octet()
|
protected |
Returns the next ready-for-shift byte, adjusting the number of parsed bytes. The larger 32-bit return type helps callers shift/merge octets into numbers. This internal method does not perform out-of-bounds checks.
Definition at line 100 of file BinaryTokenizer.cc.
◆ parsed()
|
inline |
Definition at line 105 of file BinaryTokenizer.h.
References parsed_.
Referenced by ProxyProtocol::Two::Parse(), and Parser::BinaryTokenizerContext::success().
◆ pstring16()
SBuf Parser::BinaryTokenizer::pstring16 | ( | const char * | description | ) |
Definition at line 226 of file BinaryTokenizer.cc.
Referenced by Security::Extension::Extension(), Security::TLSPlaintext::TLSPlaintext(), ProxyProtocol::Two::Parse(), Security::HandshakeParser::parseClientHelloHandshakeMessage(), Security::HandshakeParser::parseExtensions(), Security::HandshakeParser::parseServerHelloHandshakeMessage(), and Security::HandshakeParser::parseSniExtension().
◆ pstring24()
SBuf Parser::BinaryTokenizer::pstring24 | ( | const char * | description | ) |
Definition at line 235 of file BinaryTokenizer.cc.
Referenced by Security::Handshake::Handshake().
◆ pstring8()
SBuf Parser::BinaryTokenizer::pstring8 | ( | const char * | description | ) |
Definition at line 217 of file BinaryTokenizer.cc.
Referenced by Security::HandshakeParser::parseClientHelloHandshakeMessage(), Security::HandshakeParser::parseServerHelloHandshakeMessage(), and Security::HandshakeParser::parseSupportedVersionsExtension().
◆ reinput()
|
inline |
change input state without changing parsing state this method avoids append overheads during incremental parsing
Definition at line 61 of file BinaryTokenizer.h.
References data_, and expectMore_.
◆ reset()
void Parser::BinaryTokenizer::reset | ( | const SBuf & | data, |
const bool | expectMore | ||
) |
restart parsing from the very beginning this method is for using one BinaryTokenizer to parse independent inputs
Definition at line 108 of file BinaryTokenizer.cc.
◆ rollback()
void Parser::BinaryTokenizer::rollback | ( | ) |
Definition at line 114 of file BinaryTokenizer.cc.
◆ skip()
void Parser::BinaryTokenizer::skip | ( | uint64_t | size, |
const char * | description | ||
) |
Definition at line 204 of file BinaryTokenizer.cc.
References size.
Referenced by Security::HandshakeParser::parseServerHelloHandshakeMessage(), and Security::HandshakeParser::parseVersion2HandshakeMessage().
◆ skipped()
|
protected |
Definition at line 90 of file BinaryTokenizer.cc.
References BinaryTokenizer_tail, debugs, and size.
◆ uint16()
uint16_t Parser::BinaryTokenizer::uint16 | ( | const char * | description | ) |
Definition at line 141 of file BinaryTokenizer.cc.
Referenced by Security::Extension::Extension(), Security::Sslv2Record::Sslv2Record(), Security::HandshakeParser::isSslv2Record(), Security::HandshakeParser::parseCiphers(), Security::HandshakeParser::parseServerHelloHandshakeMessage(), Security::HandshakeParser::parseV23Ciphers(), and Security::HandshakeParser::parseVersion2HandshakeMessage().
◆ uint24()
uint32_t Parser::BinaryTokenizer::uint24 | ( | const char * | description | ) |
Definition at line 150 of file BinaryTokenizer.cc.
◆ uint32()
uint32_t Parser::BinaryTokenizer::uint32 | ( | const char * | description | ) |
Definition at line 159 of file BinaryTokenizer.cc.
◆ uint8()
uint8_t Parser::BinaryTokenizer::uint8 | ( | const char * | description | ) |
Definition at line 132 of file BinaryTokenizer.cc.
Referenced by Security::Alert::Alert(), Security::Handshake::Handshake(), Security::TLSPlaintext::TLSPlaintext(), Security::HandshakeParser::isSslv2Record(), ProxyProtocol::Two::Parse(), Security::HandshakeParser::parseCompressionMethods(), Security::ParseProtocolVersionBase(), Security::HandshakeParser::parseServerHelloHandshakeMessage(), Security::HandshakeParser::parseSniExtension(), Security::HandshakeParser::parseV23Ciphers(), and Security::HandshakeParser::parseVersion2HandshakeMessage().
◆ want()
|
protected |
Definition at line 45 of file BinaryTokenizer.cc.
References BinaryTokenizer_tail, debugs, Must, and size.
Member Data Documentation
◆ context
const BinaryTokenizerContext* Parser::BinaryTokenizer::context |
Definition at line 113 of file BinaryTokenizer.h.
Referenced by Parser::BinaryTokenizerContext::BinaryTokenizerContext(), and Parser::BinaryTokenizerContext::close().
◆ data_
|
private |
Definition at line 127 of file BinaryTokenizer.h.
Referenced by leftovers(), and reinput().
◆ expectMore_
|
private |
Definition at line 130 of file BinaryTokenizer.h.
Referenced by reinput().
◆ parsed_
|
private |
Definition at line 128 of file BinaryTokenizer.h.
Referenced by leftovers(), and parsed().
◆ syncPoint_
|
private |
Definition at line 129 of file BinaryTokenizer.h.
The documentation for this class was generated from the following files:
- src/parser/BinaryTokenizer.h
- src/parser/BinaryTokenizer.cc