#include <PeerSelectState.h>
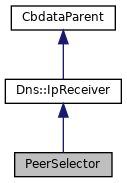
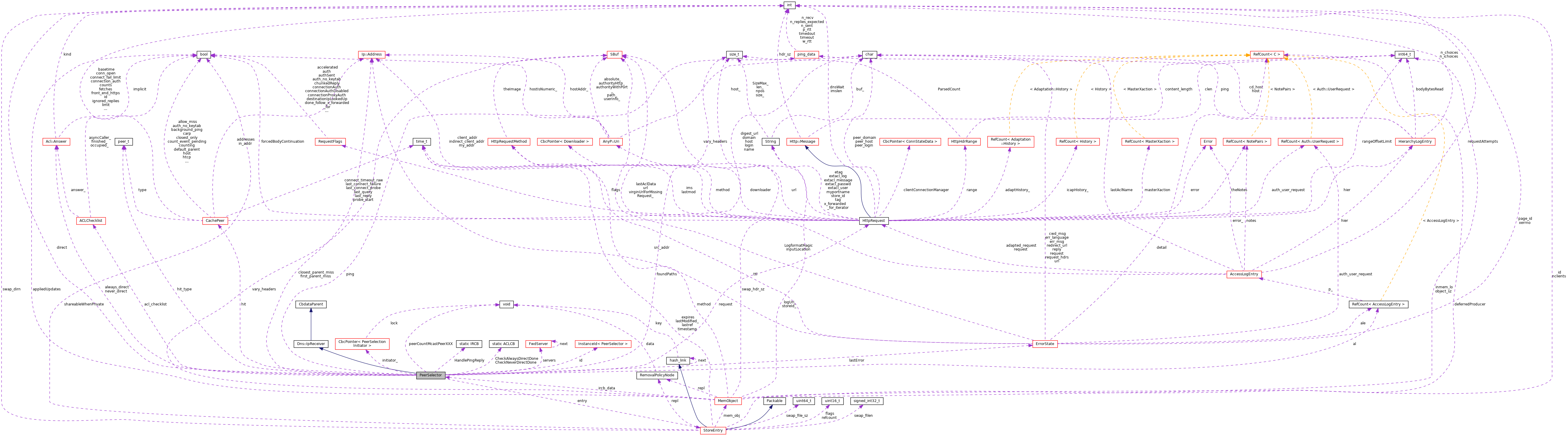
Public Member Functions | |
PeerSelector (PeerSelectionInitiator *) | |
~PeerSelector () override | |
void | noteIp (const Ip::Address &ip) override |
Called when/if nbgethostbyname() discovers a new good IP address. More... | |
void | noteIps (const Dns::CachedIps *ips, const Dns::LookupDetails &details) override |
void | noteLookup (const Dns::LookupDetails &details) override |
const SBuf | url () const |
PeerSelectionInitiator * | interestedInitiator () |
bool | wantsMoreDestinations () const |
void | handlePath (const Comm::ConnectionPointer &path, FwdServer &fs) |
processes a newly discovered/finalized path More... | |
void | selectMore () |
a single selection loop iteration: attempts to add more destinations More... | |
void | startPingWaiting () |
switches into the PING_WAITING state (and associated timeout monitoring) More... | |
void | cancelPingTimeoutMonitoring () |
terminates ICP ping timeout monitoring More... | |
virtual void * | toCbdata ()=0 |
Static Public Member Functions | |
static void | HandlePingTimeout (PeerSelector *) |
called when the given selector should stop expecting ICP ping responses More... | |
Public Attributes | |
HttpRequest * | request |
AccessLogEntry::Pointer | al |
info for the future access.log entry More... | |
StoreEntry * | entry |
void * | peerCountMcastPeerXXX = nullptr |
a hack to help peerCountMcastPeersStart() More... | |
ping_data | ping |
Protected Member Functions | |
bool | selectionAborted () |
void | handlePingTimeout () |
void | handleIcpReply (CachePeer *, const peer_t, icp_common_t *header) |
void | handleIcpParentMiss (CachePeer *, icp_common_t *) |
void | handleHtcpParentMiss (CachePeer *, HtcpReplyData *) |
void | handleHtcpReply (CachePeer *, const peer_t, HtcpReplyData *) |
int | checkNetdbDirect () |
void | checkAlwaysDirectDone (const Acl::Answer answer) |
void | checkNeverDirectDone (const Acl::Answer answer) |
void | selectSomeNeighbor () |
void | selectSomeNeighborReplies () |
Selects a neighbor (parent or sibling) based on ICP/HTCP replies. More... | |
void | selectSomeDirect () |
Adds a "direct" entry if the request can be forwarded to the origin server. More... | |
void | selectSomeParent () |
void | selectAllParents () |
Adds alive parents. Used as a last resort for never_direct. More... | |
void | selectPinned () |
Selects a pinned connection if it exists, is valid, and is allowed. More... | |
void | addSelection (CachePeer *, const hier_code) |
void | resolveSelected () |
A single DNS resolution loop iteration: Converts selected FwdServer to IPs. More... | |
Static Protected Attributes | |
static IRCB | HandlePingReply |
static ACLCB | CheckAlwaysDirectDone |
static ACLCB | CheckNeverDirectDone |
Private Types | |
typedef CbcPointer< PeerSelectionInitiator > | Initiator |
Private Member Functions | |
CBDATA_CHILD (PeerSelector) | |
virtual void | finalizedInCbdataChild ()=0 |
hack: ensure CBDATA_CHILD() after a toCbdata()-defining CBDATA_INTERMEDIATE() More... | |
Private Attributes | |
Acl::Answer | always_direct |
Acl::Answer | never_direct |
int | direct |
size_t | foundPaths = 0 |
number of unique destinations identified so far More... | |
ErrorState * | lastError |
FwdServer * | servers |
a linked list of (unresolved) selected peers More... | |
Ip::Address | first_parent_miss |
Ip::Address | closest_parent_miss |
CachePeer * | hit |
peer_t | hit_type |
ACLChecklist * | acl_checklist |
Initiator | initiator_ |
recipient of the destinations we select; use interestedInitiator() to access More... | |
const InstanceId< PeerSelector > | id |
unique identification in worker log More... | |
Detailed Description
Finds peer (including origin server) IPs for forwarding a single request. Gives PeerSelectionInitiator each found destination, in the right order.
Definition at line 59 of file PeerSelectState.h.
Member Typedef Documentation
◆ Initiator
|
private |
Definition at line 163 of file PeerSelectState.h.
Constructor & Destructor Documentation
◆ PeerSelector()
|
explicit |
Definition at line 1110 of file peer_select.cc.
◆ ~PeerSelector()
|
override |
Definition at line 231 of file peer_select.cc.
References acl_checklist, assert, cancelPingTimeoutMonitoring(), DBG_IMPORTANT, debugs, entry, HTTPMSGUNLOCK(), lastError, FwdServer::next, PING_DONE, StoreEntry::ping_status, PING_WAITING, request, servers, StoreEntry::unlock(), and StoreEntry::url().
Member Function Documentation
◆ addSelection()
Definition at line 1086 of file peer_select.cc.
References code, debugs, PINNED, server, and servers.
Referenced by selectAllParents(), selectPinned(), selectSomeDirect(), selectSomeNeighbor(), selectSomeNeighborReplies(), and selectSomeParent().
◆ cancelPingTimeoutMonitoring()
void PeerSelector::cancelPingTimeoutMonitoring | ( | ) |
Definition at line 272 of file peer_select.cc.
References PeerSelectorPingMonitor::forget(), and PingMonitor().
Referenced by ~PeerSelector(), and selectMore().
◆ CBDATA_CHILD()
|
private |
◆ checkAlwaysDirectDone()
|
protected |
if always_direct says YES, do that.
Definition at line 371 of file peer_select.cc.
References ACCESS_ALLOWED, ACCESS_AUTH_REQUIRED, ACCESS_DENIED, ACCESS_DUNNO, acl_checklist, always_direct, DBG_IMPORTANT, debugs, direct, DIRECT_YES, DirectStr, and selectMore().
◆ checkNetdbDirect()
|
protected |
Definition at line 568 of file peer_select.cc.
References closest_parent_miss, Config, debugs, direct, DIRECT_NO, AnyP::Uri::host(), SquidConfig::minDirectHops, SquidConfig::minDirectRtt, netdbHostHops(), netdbHostRtt(), ping_data::p_rtt, ping, request, HttpRequest::url, and whichPeer().
Referenced by selectMore(), and selectSomeNeighborReplies().
◆ checkNeverDirectDone()
|
protected |
if never_direct says YES, do that.
Definition at line 343 of file peer_select.cc.
References ACCESS_ALLOWED, ACCESS_AUTH_REQUIRED, ACCESS_DENIED, ACCESS_DUNNO, acl_checklist, DBG_IMPORTANT, debugs, direct, DIRECT_NO, DirectStr, never_direct, and selectMore().
◆ finalizedInCbdataChild()
|
privatepure virtualinherited |
◆ handleHtcpParentMiss()
|
protected |
Definition at line 1026 of file peer_select.cc.
References CachePeer::basetime, CachePeer::closest_only, closest_parent_miss, Config, HtcpReplyData::cto, current_time, first_parent_miss, HtcpReplyData::cto_t::hops, CachePeer::in_addr, int, Ip::Address::isAnyAddr(), netdbUpdatePeer(), SquidConfig::onoff, CachePeer::options, ping_data::p_rtt, ping, SquidConfig::query_icmp, request, HtcpReplyData::cto_t::rtt, ping_data::start, tvSubMsec(), HttpRequest::url, ping_data::w_rtt, and CachePeer::weight.
Referenced by handleHtcpReply().
◆ handleHtcpReply()
|
protected |
Definition at line 1004 of file peer_select.cc.
References debugs, handleHtcpParentMiss(), HtcpReplyData::hit, hit, hit_type, ping_data::n_recv, ping_data::n_replies_expected, PEER_PARENT, ping, selectMore(), and url().
◆ handleIcpParentMiss()
|
protected |
Definition at line 928 of file peer_select.cc.
References CachePeer::basetime, CachePeer::closest_only, closest_parent_miss, Config, current_time, first_parent_miss, icp_common_t::flags, ICP_FLAG_SRC_RTT, CachePeer::in_addr, Ip::Address::isAnyAddr(), netdbUpdatePeer(), SquidConfig::onoff, CachePeer::options, ping_data::p_rtt, icp_common_t::pad, ping, SquidConfig::query_icmp, request, ping_data::start, tvSubMsec(), HttpRequest::url, ping_data::w_rtt, and CachePeer::weight.
Referenced by handleIcpReply().
◆ handleIcpReply()
|
protected |
Definition at line 971 of file peer_select.cc.
References debugs, icp_common_t::getOpCode(), handleIcpParentMiss(), hit, hit_type, ICP_DECHO, ICP_HIT, ICP_MISS, icp_opcode_str, ping_data::n_recv, ping_data::n_replies_expected, PEER_PARENT, peerDigestLookup(), peerNoteDigestLookup(), ping, request, selectMore(), and url().
◆ handlePath()
void PeerSelector::handlePath | ( | const Comm::ConnectionPointer & | path, |
FwdServer & | fs | ||
) |
Definition at line 1168 of file peer_select.cc.
References FwdServer::_peer, always_direct, FwdServer::code, debugs, foundPaths, CbcPointer< Cbc >::get(), getOutgoingAddress(), HttpRequest::hier, interestedInitiator(), never_direct, Comm::Connection::peerType, HierarchyLogEntry::ping, ping, request, Comm::Connection::setPeer(), ping_data::timedout, and url().
Referenced by noteIp(), and resolveSelected().
◆ handlePingTimeout()
|
protected |
Definition at line 897 of file peer_select.cc.
References debugs, entry, PeerStats, ping, PING_DONE, StoreEntry::ping_status, PING_WAITING, selectionAborted(), selectMore(), ping_data::timedout, and url().
Referenced by HandlePingTimeout().
◆ HandlePingTimeout()
|
static |
Definition at line 916 of file peer_select.cc.
References handlePingTimeout().
◆ interestedInitiator()
PeerSelectionInitiator * PeerSelector::interestedInitiator | ( | ) |
- Returns
- valid/interested peer initiator or nil
Definition at line 1143 of file peer_select.cc.
References debugs, initiator_, and CbcPointer< Cbc >::valid().
Referenced by handlePath(), resolveSelected(), and selectionAborted().
◆ noteIp()
|
overridevirtual |
Reimplemented from Dns::IpReceiver.
Definition at line 516 of file peer_select.cc.
References FwdServer::_peer, HttpRequest::client_addr, HttpRequest::flags, handlePath(), Ip::Address::isIPv4(), Ip::Address::port(), AnyP::Uri::port(), Comm::Connection::remote, request, selectionAborted(), servers, RequestFlags::spoofClientIp, HttpRequest::url, CbcPointer< Cbc >::valid(), and wantsMoreDestinations().
◆ noteIps()
|
overridevirtual |
Called when nbgethostbyname() fully resolves the name. The ips
may contain both bad and good IP addresses, but each good IP (if any) is guaranteed to had been previously reported via noteIp(). When no IPs were obtained, ips
is nil.
Implements Dns::IpReceiver.
Definition at line 542 of file peer_select.cc.
References FwdServer::_peer, al, FwdServer::code, debugs, ErrorState::dnsError, ERR_DNS_FAIL, Dns::LookupDetails::error, HIER_DIRECT, AnyP::Uri::host(), CachePeer::host, lastError, FwdServer::next, request, resolveSelected(), Http::scServiceUnavailable, selectionAborted(), servers, HttpRequest::url, and CbcPointer< Cbc >::valid().
◆ noteLookup()
|
overridevirtual |
Called when/if nbgethostbyname() completes a single DNS lookup if called, called before all the noteIp() calls for that DNS lookup.
Reimplemented from Dns::IpReceiver.
Definition at line 502 of file peer_select.cc.
References HttpRequest::recordLookup(), request, selectionAborted(), and wantsMoreDestinations().
◆ resolveSelected()
|
protected |
Definition at line 413 of file peer_select.cc.
References FwdServer::_peer, always_direct, assert, SquidConfig::client_dst_passthru, Server::clientConnection, HttpRequest::clientConnectionManager, FwdServer::code, Config, current_time, debugs, HttpRequest::flags, foundPaths, handlePath(), HttpRequest::hier, HIER_DIRECT, AnyP::Uri::host(), CachePeer::host, RequestFlags::hostVerified, RequestFlags::intercepted, RequestFlags::interceptTproxy, interestedInitiator(), lastError, Comm::Connection::local, Dns::nbgethostbyname(), never_direct, FwdServer::next, SquidConfig::onoff, ORIGINAL_DST, HierarchyLogEntry::ping, ping, PINNED, RequestFlags::redirected, Comm::Connection::remote, request, resolveSelected(), selectionAborted(), servers, ping_data::stop, ping_data::timedout, HttpRequest::url, url(), CbcPointer< Cbc >::valid(), and wantsMoreDestinations().
Referenced by noteIps(), resolveSelected(), and selectMore().
◆ selectAllParents()
|
protected |
Definition at line 867 of file peer_select.cc.
References addSelection(), ANY_OLD_PARENT, CurrentCachePeers(), DEFAULT_PARENT, getDefaultParent(), neighborType(), PEER_PARENT, peerHTTPOkay(), request, and HttpRequest::url.
Referenced by selectMore().
◆ selectionAborted()
|
protected |
- Returns
- true (after destroying "this") if the peer initiator is gone
- false (without side effects) otherwise
Definition at line 401 of file peer_select.cc.
References debugs, and interestedInitiator().
Referenced by handlePingTimeout(), noteIp(), noteIps(), noteLookup(), resolveSelected(), and selectMore().
◆ selectMore()
void PeerSelector::selectMore | ( | ) |
If we don't know whether DIRECT is permitted ...
check always_direct;
check never_direct;
if we are accelerating, direct is not an option.
if we are in a forwarding-loop, direct is not an option.
Definition at line 611 of file peer_select.cc.
References ACCESS_DUNNO, SquidConfig::accessList, acl_checklist, ACLFilledChecklist::al, al, always_direct, SquidConfig::AlwaysDirect, cancelPingTimeoutMonitoring(), CheckAlwaysDirectDone, checkNetdbDirect(), CheckNeverDirectDone, Config, debugs, direct, DIRECT_MAYBE, DIRECT_NO, DIRECT_UNKNOWN, DIRECT_YES, DirectStr, entry, HttpRequest::flags, RequestFlags::hierarchical, AnyP::Uri::host(), RequestFlags::loopDetected, HttpRequest::method, never_direct, SquidConfig::NeverDirect, RequestFlags::noDirect, ACLChecklist::nonBlockingCheck(), SquidConfig::nonhierarchical_direct, SquidConfig::onoff, PING_DONE, PING_NONE, StoreEntry::ping_status, PING_WAITING, SquidConfig::prefer_direct, request, resolveSelected(), selectAllParents(), selectionAborted(), selectPinned(), selectSomeDirect(), selectSomeNeighbor(), selectSomeNeighborReplies(), selectSomeParent(), ACLChecklist::syncAle(), and HttpRequest::url.
Referenced by checkAlwaysDirectDone(), checkNeverDirectDone(), handleHtcpReply(), handleIcpReply(), and handlePingTimeout().
◆ selectPinned()
|
protected |
Definition at line 707 of file peer_select.cc.
References addSelection(), direct, DIRECT_NO, entry, ConnStateData::peerAccessDenied, peerAllowedToUse(), PING_DONE, StoreEntry::ping_status, PINNED, HttpRequest::pinnedConnection(), ConnStateData::pinnedPeer(), ConnStateData::pinning, and request.
Referenced by selectMore().
◆ selectSomeDirect()
|
protected |
Definition at line 820 of file peer_select.cc.
References addSelection(), direct, DIRECT_NO, AnyP::Uri::getScheme(), HIER_DIRECT, AnyP::PROTO_WAIS, request, and HttpRequest::url.
Referenced by selectMore().
◆ selectSomeNeighbor()
|
protected |
Selects a neighbor (parent or sibling) based on one of the following methods: Cache Digests CARP ICMP Netdb RTT estimates ICP/HTCP queries
Definition at line 733 of file peer_select.cc.
References addSelection(), assert, CD_PARENT_HIT, CD_SIBLING_HIT, CLOSEST_PARENT, code, current_time, DBG_CRITICAL, debugs, direct, DIRECT_YES, entry, HandlePingReply, HIER_NONE, ping_data::n_replies_expected, ping_data::n_sent, neighborsDigestSelect(), neighborsUdpPing(), neighborType(), netdbClosestParent(), PEER_PARENT, peerSelectIcpPing(), ping, PING_DONE, PING_NONE, StoreEntry::ping_status, request, ping_data::start, startPingWaiting(), ping_data::timeout, and HttpRequest::url.
Referenced by selectMore().
◆ selectSomeNeighborReplies()
|
protected |
Definition at line 789 of file peer_select.cc.
References addSelection(), assert, checkNetdbDirect(), CLOSEST_DIRECT, CLOSEST_PARENT_MISS, closest_parent_miss, code, direct, DIRECT_YES, entry, FIRST_PARENT_MISS, first_parent_miss, HIER_NONE, hit, hit_type, Ip::Address::isAnyAddr(), PARENT_HIT, PEER_PARENT, StoreEntry::ping_status, PING_WAITING, SIBLING_HIT, and whichPeer().
Referenced by selectMore().
◆ selectSomeParent()
|
protected |
Definition at line 833 of file peer_select.cc.
References addSelection(), CARP, carpSelectParent(), code, debugs, DEFAULT_PARENT, direct, DIRECT_YES, FIRSTUP_PARENT, getDefaultParent(), getFirstUpParent(), getRoundRobinParent(), getWeightedRoundRobinParent(), HIER_NONE, AnyP::Uri::host(), HttpRequest::method, peerSourceHashSelectParent(), peerUserHashSelectParent(), request, ROUNDROBIN_PARENT, SOURCEHASH_PARENT, HttpRequest::url, and USERHASH_PARENT.
Referenced by selectMore().
◆ startPingWaiting()
void PeerSelector::startPingWaiting | ( | ) |
Definition at line 263 of file peer_select.cc.
References assert, entry, PeerSelectorPingMonitor::monitor(), StoreEntry::ping_status, PING_WAITING, and PingMonitor().
Referenced by selectSomeNeighbor().
◆ toCbdata()
|
pure virtualinherited |
Referenced by AsyncJob::callException(), and AsyncJob::callStart().
◆ url()
const SBuf PeerSelector::url | ( | ) | const |
Definition at line 1129 of file peer_select.cc.
References HttpRequest::effectiveRequestUri(), entry, request, and StoreEntry::url().
Referenced by handleHtcpReply(), handleIcpReply(), handlePath(), handlePingTimeout(), and resolveSelected().
◆ wantsMoreDestinations()
bool PeerSelector::wantsMoreDestinations | ( | ) | const |
- Returns
- whether the initiator may use more destinations
Definition at line 1162 of file peer_select.cc.
References Config, and SquidConfig::forward_max_tries.
Referenced by noteIp(), noteLookup(), and resolveSelected().
Member Data Documentation
◆ acl_checklist
|
private |
Definition at line 161 of file PeerSelectState.h.
Referenced by ~PeerSelector(), checkAlwaysDirectDone(), checkNeverDirectDone(), and selectMore().
◆ al
AccessLogEntry::Pointer PeerSelector::al |
Definition at line 98 of file PeerSelectState.h.
Referenced by noteIps(), peerAllowedToUse(), and selectMore().
◆ always_direct
|
private |
Definition at line 136 of file PeerSelectState.h.
Referenced by checkAlwaysDirectDone(), handlePath(), resolveSelected(), and selectMore().
◆ CheckAlwaysDirectDone
|
staticprotected |
Definition at line 132 of file PeerSelectState.h.
Referenced by selectMore().
◆ CheckNeverDirectDone
|
staticprotected |
Definition at line 133 of file PeerSelectState.h.
Referenced by selectMore().
◆ closest_parent_miss
|
private |
Definition at line 154 of file PeerSelectState.h.
Referenced by checkNetdbDirect(), handleHtcpParentMiss(), handleIcpParentMiss(), and selectSomeNeighborReplies().
◆ direct
|
private |
Definition at line 138 of file PeerSelectState.h.
Referenced by checkAlwaysDirectDone(), checkNetdbDirect(), checkNeverDirectDone(), selectMore(), selectPinned(), selectSomeDirect(), selectSomeNeighbor(), selectSomeNeighborReplies(), and selectSomeParent().
◆ entry
StoreEntry* PeerSelector::entry |
Definition at line 99 of file PeerSelectState.h.
Referenced by ~PeerSelector(), handlePingTimeout(), peerCountMcastPeersAbort(), selectMore(), selectPinned(), selectSomeNeighbor(), selectSomeNeighborReplies(), startPingWaiting(), and url().
◆ first_parent_miss
|
private |
Definition at line 152 of file PeerSelectState.h.
Referenced by handleHtcpParentMiss(), handleIcpParentMiss(), and selectSomeNeighborReplies().
◆ foundPaths
|
private |
Definition at line 139 of file PeerSelectState.h.
Referenced by handlePath(), and resolveSelected().
◆ HandlePingReply
|
staticprotected |
Definition at line 131 of file PeerSelectState.h.
Referenced by selectSomeNeighbor().
◆ hit
|
private |
Definition at line 159 of file PeerSelectState.h.
Referenced by handleHtcpReply(), handleIcpReply(), and selectSomeNeighborReplies().
◆ hit_type
|
private |
Definition at line 160 of file PeerSelectState.h.
Referenced by handleHtcpReply(), handleIcpReply(), and selectSomeNeighborReplies().
◆ id
|
private |
Definition at line 166 of file PeerSelectState.h.
◆ initiator_
|
private |
Definition at line 164 of file PeerSelectState.h.
Referenced by interestedInitiator().
◆ lastError
|
private |
Definition at line 140 of file PeerSelectState.h.
Referenced by ~PeerSelector(), noteIps(), and resolveSelected().
◆ never_direct
|
private |
Definition at line 137 of file PeerSelectState.h.
Referenced by checkNeverDirectDone(), handlePath(), resolveSelected(), and selectMore().
◆ peerCountMcastPeerXXX
void* PeerSelector::peerCountMcastPeerXXX = nullptr |
Definition at line 101 of file PeerSelectState.h.
Referenced by peerCountMcastPeersAbort().
◆ ping
ping_data PeerSelector::ping |
Definition at line 103 of file PeerSelectState.h.
Referenced by checkNetdbDirect(), PeerSelectorPingMonitor::forget(), handleHtcpParentMiss(), handleHtcpReply(), handleIcpParentMiss(), handleIcpReply(), handlePath(), handlePingTimeout(), PeerSelectorPingMonitor::monitor(), peerCountMcastPeersAbort(), resolveSelected(), and selectSomeNeighbor().
◆ request
HttpRequest* PeerSelector::request |
Definition at line 97 of file PeerSelectState.h.
Referenced by ~PeerSelector(), carpSelectParent(), checkNetdbDirect(), getDefaultParent(), getFirstUpParent(), getRoundRobinParent(), getWeightedRoundRobinParent(), handleHtcpParentMiss(), handleIcpParentMiss(), handleIcpReply(), handlePath(), neighborsDigestSelect(), netdbClosestParent(), noteIp(), noteIps(), noteLookup(), operator<<(), peerAllowedToUse(), peerDigestLookup(), peerSelectIcpPing(), peerSourceHashSelectParent(), peerUserHashSelectParent(), peerWouldBePinged(), resolveSelected(), selectAllParents(), selectMore(), selectPinned(), selectSomeDirect(), selectSomeNeighbor(), selectSomeParent(), and url().
◆ servers
|
private |
Definition at line 142 of file PeerSelectState.h.
Referenced by ~PeerSelector(), addSelection(), noteIp(), noteIps(), and resolveSelected().
The documentation for this class was generated from the following files:
- src/PeerSelectState.h
- src/peer_select.cc