#include "squid.h"
#include "base/PackableStream.h"
#include "HttpHdrCc.h"
#include "HttpReply.h"
#include "HttpRequest.h"
#include "MemObject.h"
#include "mgr/Registration.h"
#include "refresh.h"
#include "RefreshPattern.h"
#include "SquidConfig.h"
#include "Store.h"
#include "util.h"
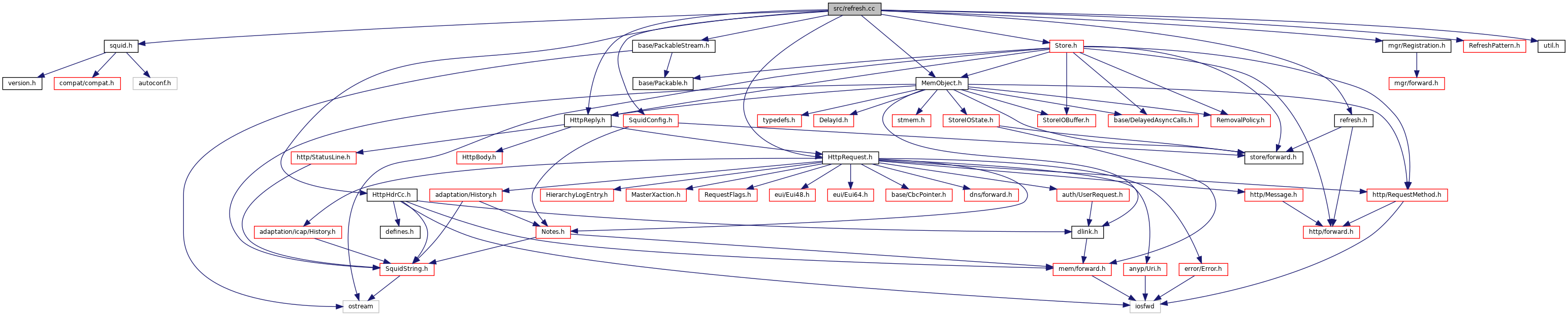
Go to the source code of this file.
Classes | |
struct | stale_flags |
struct | RefreshCounts |
Macros | |
#define | USE_POSIX_REGEX /* put before includes; always use POSIX */ |
Enumerations | |
enum | refreshCountsEnum { rcHTTP , rcICP , rcHTCP , rcCDigest , rcStore , rcCount } |
enum | { FRESH_REQUEST_MAX_STALE_ALL = 100 , FRESH_REQUEST_MAX_STALE_VALUE , FRESH_EXPIRES , FRESH_LMFACTOR_RULE , FRESH_MIN_RULE , FRESH_OVERRIDE_EXPIRES , FRESH_OVERRIDE_LASTMOD , STALE_MUST_REVALIDATE = 200 , STALE_RELOAD_INTO_IMS , STALE_FORCED_RELOAD , STALE_EXCEEDS_REQUEST_MAX_AGE_VALUE , STALE_EXPIRES , STALE_MAX_RULE , STALE_LMFACTOR_RULE , STALE_MAX_STALE , STALE_DEFAULT = 299 } |
Functions | |
static int | refreshStaleness (const StoreEntry *entry, time_t check_time, const time_t age, const RefreshPattern *R, stale_flags *sf) |
const RefreshPattern * | refreshLimits (const char *url) |
static const RefreshPattern * | refreshFirstDotRule () |
the first explicit refresh_pattern rule that uses a "." regex (or nil) More... | |
static int | refreshCheck (const StoreEntry *entry, HttpRequest *request, time_t delta) |
bool | refreshIsCachable (const StoreEntry *entry) |
static bool | refreshIsStaleIfHit (const int reason) |
whether reply is stale if it is a hit More... | |
int | refreshCheckHTTP (const StoreEntry *entry, HttpRequest *request) |
int | refreshCheckICP (const StoreEntry *entry, HttpRequest *request) |
int | refreshCheckHTCP (const StoreEntry *entry, HttpRequest *request) |
int | refreshCheckDigest (const StoreEntry *entry, time_t delta) |
time_t | getMaxAge (const char *url) |
static int | refreshCountsStatsEntry (StoreEntry *sentry, struct RefreshCounts &rc, int code, const char *desc) |
static void | refreshCountsStats (StoreEntry *sentry, struct RefreshCounts &rc) |
static void | refreshStats (StoreEntry *sentry) |
static void | refreshRegisterWithCacheManager (void) |
void | refreshInit (void) |
Variables | |
static struct RefreshCounts | refreshCounts [rcCount] |
static OBJH | refreshStats |
static RefreshPattern | DefaultRefresh (nullptr) |
Macro Definition Documentation
◆ USE_POSIX_REGEX
#define USE_POSIX_REGEX /* put before includes; always use POSIX */ |
Definition at line 12 of file refresh.cc.
Enumeration Type Documentation
◆ anonymous enum
anonymous enum |
Definition at line 57 of file refresh.cc.
◆ refreshCountsEnum
enum refreshCountsEnum |
Enumerator | |
---|---|
rcHTTP | |
rcICP | |
rcHTCP | |
rcCDigest | |
rcStore | |
rcCount |
Definition at line 28 of file refresh.cc.
Function Documentation
◆ getMaxAge()
time_t getMaxAge | ( | const char * | url | ) |
Get the configured maximum caching time for objects with this URL according to refresh_pattern.
Used by http.cc when generating a upstream requests to ensure that responses it is given are fresh enough to be worth caching.
- Return values
-
pattern-max if there is a refresh_pattern matching the URL configured. REFRESH_DEFAULT_MAX if there are no explicit limits configured
Definition at line 633 of file refresh.cc.
References debugs, RefreshPattern::max, REFRESH_DEFAULT_MAX, and refreshLimits().
Referenced by HttpStateData::httpBuildRequestHeader().
◆ refreshCheck()
|
static |
Checks whether a store entry is fresh or stale, and why.
This is where all aspects of request, response and squid configuration meet to decide whether a response is cacheable or not:
- Client request headers that affect cacheability, e.g.
- Cache-Control: no-cache
- Cache-Control: max-age=N
- Cache-Control: max-stale[=N]
- Pragma: no-cache
- Server response headers that affect cacheability, e.g.
- Age:
- Cache-Control: proxy-revalidate
- Cache-Control: must-revalidate
- Cache-Control: no-cache
- Cache-Control: max-age=N
- Cache-Control: s-maxage=N
- Date:
- Expires:
- Last-Modified:
- Configuration options, e.g.
- reload-into-ims (refresh_pattern)
- ignore-reload (refresh_pattern)
- refresh-ims (refresh_pattern)
- override-lastmod (refresh_pattern)
- override-expire (refresh_pattern)
- reload_into_ims (global option)
- refresh_all_ims (global option)
- Returns
- a status code (from enum above):
- FRESH_REQUEST_MAX_STALE_ALL
- FRESH_REQUEST_MAX_STALE_VALUE
- FRESH_EXPIRES
- FRESH_LMFACTOR_RULE
- FRESH_MIN_RULE
- FRESH_OVERRIDE_EXPIRES
- FRESH_OVERRIDE_LASTMOD
- STALE_MUST_REVALIDATE
- STALE_RELOAD_INTO_IMS
- STALE_FORCED_RELOAD
- STALE_EXCEEDS_REQUEST_MAX_AGE_VALUE
- STALE_EXPIRES
- STALE_MAX_RULE
- STALE_LMFACTOR_RULE
- STALE_MAX_STALE
- STALE_DEFAULT
- Note
- request may be NULL (e.g. for cache digests build)
- the store entry being examined is not necessarily cached (e.g. if this response is being evaluated for the first time)
Definition at line 257 of file refresh.cc.
References assert, SBuf::c_str(), Http::Message::cache_control, Config, debugs, DefaultRefresh, EBIT_TEST, HttpRequest::effectiveRequestUri(), ENTRY_REVALIDATE_ALWAYS, ENTRY_REVALIDATE_STALE, stale_flags::expires, RequestFlags::failOnValidationError, HttpRequest::flags, RefreshPattern::flags, StoreEntry::flags, Time::FormatRfc1123(), FRESH_EXPIRES, FRESH_LMFACTOR_RULE, FRESH_MIN_RULE, FRESH_OVERRIDE_EXPIRES, FRESH_OVERRIDE_LASTMOD, FRESH_REQUEST_MAX_STALE_ALL, FRESH_REQUEST_MAX_STALE_VALUE, StoreEntry::hasFreshestReply(), HttpHdrCc::hasMaxAge(), HttpHdrCc::hasMaxStale(), HttpHdrCc::hasMinFresh(), HttpHdrCc::hasStaleIfError(), RefreshPattern::ignore_reload, RequestFlags::ignoreCc, RequestFlags::ims, stale_flags::lmfactor, stale_flags::max, RefreshPattern::max_stale, HttpHdrCc::MAX_STALE_ANY, SquidConfig::maxStale, StoreEntry::mem_obj, min(), stale_flags::min, RequestFlags::noCache, RequestFlags::noCacheHack(), SquidConfig::onoff, RefreshPattern::override_expire, RefreshPattern::override_lastmod, SquidConfig::refresh_all_ims, RefreshPattern::refresh_ims, refreshFirstDotRule(), refreshLimits(), refreshStaleness(), RefreshPattern::reload_into_ims, SquidConfig::reload_into_ims, squid_curtime, STALE_DEFAULT, STALE_EXCEEDS_REQUEST_MAX_AGE_VALUE, STALE_EXPIRES, STALE_FORCED_RELOAD, STALE_LMFACTOR_RULE, STALE_MAX_RULE, STALE_MAX_STALE, STALE_MUST_REVALIDATE, STALE_RELOAD_INTO_IMS, MemObject::storeId(), and StoreEntry::timestamp.
Referenced by refreshCheckDigest(), refreshCheckHTCP(), refreshCheckHTTP(), refreshCheckICP(), and refreshIsCachable().
◆ refreshCheckDigest()
int refreshCheckDigest | ( | const StoreEntry * | entry, |
time_t | delta | ||
) |
Definition at line 611 of file refresh.cc.
References RefCount< C >::getRaw(), StoreEntry::mem_obj, rcCDigest, refreshCheck(), refreshCounts, MemObject::request, RefreshCounts::status, and RefreshCounts::total.
Referenced by storeDigestAddable().
◆ refreshCheckHTCP()
int refreshCheckHTCP | ( | const StoreEntry * | entry, |
HttpRequest * | request | ||
) |
Definition at line 598 of file refresh.cc.
References rcHTCP, refreshCheck(), refreshCounts, RefreshCounts::status, and RefreshCounts::total.
Referenced by htcpSpecifier::checkHit().
◆ refreshCheckHTTP()
int refreshCheckHTTP | ( | const StoreEntry * | entry, |
HttpRequest * | request | ||
) |
Protocol-specific wrapper around refreshCheck() function.
Note the reason for STALE/FRESH then return true/false respectively.
- Return values
-
1 if STALE 0 if FRESH
Definition at line 575 of file refresh.cc.
References Config, HttpRequest::flags, SquidConfig::offline, SquidConfig::onoff, rcHTTP, refreshCheck(), refreshCounts, refreshIsStaleIfHit(), RequestFlags::staleIfHit, RefreshCounts::status, and RefreshCounts::total.
Referenced by clientReplyContext::cacheHit().
◆ refreshCheckICP()
int refreshCheckICP | ( | const StoreEntry * | entry, |
HttpRequest * | request | ||
) |
Definition at line 587 of file refresh.cc.
References rcICP, refreshCheck(), refreshCounts, RefreshCounts::status, and RefreshCounts::total.
Referenced by ICPState::confirmAndPrepHit().
◆ refreshCountsStats()
|
static |
Definition at line 652 of file refresh.cc.
References FRESH_EXPIRES, FRESH_LMFACTOR_RULE, FRESH_MIN_RULE, FRESH_OVERRIDE_EXPIRES, FRESH_OVERRIDE_LASTMOD, FRESH_REQUEST_MAX_STALE_ALL, FRESH_REQUEST_MAX_STALE_VALUE, RefreshCounts::proto, refreshCountsStatsEntry(), STALE_DEFAULT, STALE_EXCEEDS_REQUEST_MAX_AGE_VALUE, STALE_EXPIRES, STALE_FORCED_RELOAD, STALE_LMFACTOR_RULE, STALE_MAX_RULE, STALE_MUST_REVALIDATE, STALE_RELOAD_INTO_IMS, storeAppendPrintf(), and RefreshCounts::total.
Referenced by refreshStats().
◆ refreshCountsStatsEntry()
|
static |
Definition at line 645 of file refresh.cc.
References code, RefreshCounts::status, storeAppendPrintf(), RefreshCounts::total, and xpercent().
Referenced by refreshCountsStats().
◆ refreshFirstDotRule()
|
static |
Definition at line 107 of file refresh.cc.
References Config, RefreshPattern::next, and SquidConfig::Refresh.
Referenced by refreshCheck().
◆ refreshInit()
void refreshInit | ( | void | ) |
Definition at line 754 of file refresh.cc.
References RefreshCounts::proto, rcCDigest, rcHTCP, rcHTTP, rcICP, rcStore, refreshCounts, and refreshRegisterWithCacheManager().
Referenced by mainInitialize().
◆ refreshIsCachable()
bool refreshIsCachable | ( | const StoreEntry * | entry | ) |
This is called by http.cc once it has received and parsed the origin server's response headers. It uses the result as part of its algorithm to decide whether a response should be cached.
- Return values
-
true if the entry is cacheable, regardless of whether FRESH or STALE false if the entry is not cacheable
TODO: this algorithm seems a bit odd and might not be quite right. Verify against HTTPbis.
Definition at line 519 of file refresh.cc.
References MemObject::baseReply(), Config, Http::Message::content_length, StoreEntry::lastModified(), StoreEntry::mem_obj, SquidConfig::minimum_expiry_time, rcStore, refreshCheck(), refreshCounts, STALE_MUST_REVALIDATE, RefreshCounts::status, and RefreshCounts::total.
Referenced by HttpStateData::reusableReply().
◆ refreshIsStaleIfHit()
|
static |
Definition at line 554 of file refresh.cc.
References FRESH_EXPIRES, FRESH_LMFACTOR_RULE, and FRESH_MIN_RULE.
Referenced by refreshCheckHTTP().
◆ refreshLimits()
const RefreshPattern * refreshLimits | ( | const char * | url | ) |
Locate the first refresh_pattern rule that matches the given URL by regex.
- Returns
- A pointer to the refresh_pattern parameters to use, or nullptr if there is no match.
Definition at line 92 of file refresh.cc.
References Config, RefreshPattern::next, and SquidConfig::Refresh.
Referenced by getMaxAge(), and refreshCheck().
◆ refreshRegisterWithCacheManager()
|
static |
Definition at line 748 of file refresh.cc.
References refreshStats, and Mgr::RegisterAction().
Referenced by refreshInit().
◆ refreshStaleness()
|
static |
Calculate how stale the response is (or will be at the check_time).
We try the following ways until one gives a result:
- response expiration time, if one was set
- age greater than configured maximum
- last-modified factor algorithm
- age less than configured minimum
- default (stale)
- Parameters
-
entry the StoreEntry being examined check_time the time (maybe future) at which we want to know whether $ age the age of the entry at check_time R the refresh_pattern rule that matched this entry sf small struct to indicate reason for stale/fresh decision
- Return values
-
-1 If the response is fresh. >0 The amount of staleness. 0 NOTE return value of 0 means the response is stale.
Definition at line 139 of file refresh.cc.
References debugs, stale_flags::expires, StoreEntry::expires, StoreEntry::lastModified(), stale_flags::lmfactor, stale_flags::max, RefreshPattern::max, min(), stale_flags::min, RefreshPattern::min, RefreshPattern::pct, and StoreEntry::timestamp.
Referenced by refreshCheck().
◆ refreshStats()
|
static |
Definition at line 679 of file refresh.cc.
References Config, RefreshPattern::next, PRIu64, rcCount, SquidConfig::Refresh, refreshCounts, refreshCountsStats(), storeAppendPrintf(), and xpercent().
Variable Documentation
◆ DefaultRefresh
|
static |
Referenced by refreshCheck().
◆ refreshCounts
|
static |
Referenced by refreshCheckDigest(), refreshCheckHTCP(), refreshCheckHTTP(), refreshCheckICP(), refreshInit(), refreshIsCachable(), and refreshStats().
◆ refreshStats
|
static |
Definition at line 82 of file refresh.cc.
Referenced by refreshRegisterWithCacheManager().