#include <FwdState.h>
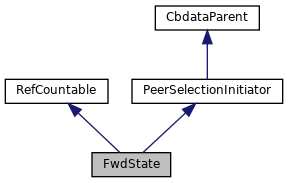
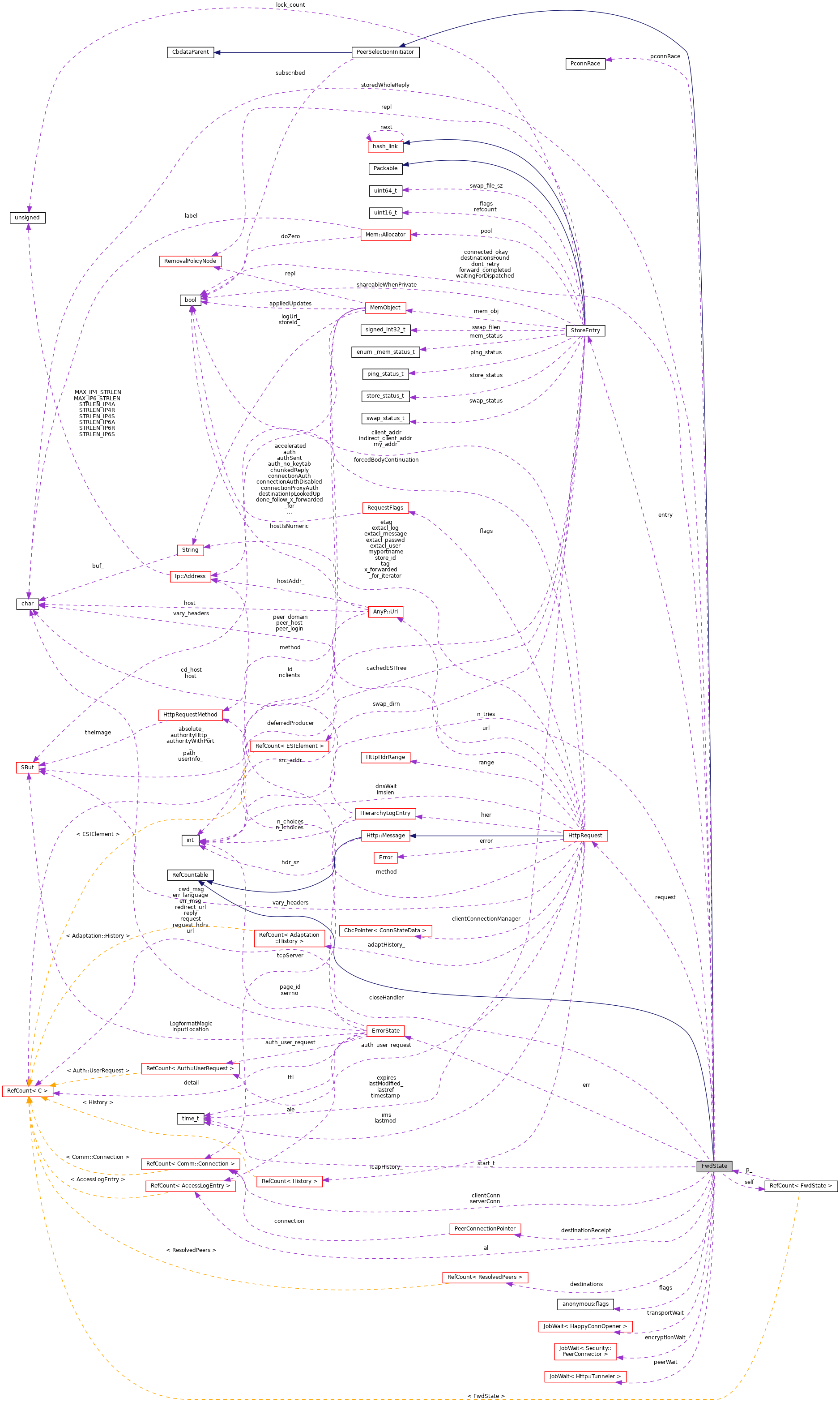
Public Types | |
typedef RefCount< FwdState > | Pointer |
Static Public Member Functions | |
static void | initModule () |
static void | Start (const Comm::ConnectionPointer &client, StoreEntry *, HttpRequest *, const AccessLogEntryPointer &alp) |
Initiates request forwarding to a peer or origin server. More... | |
static void | fwdStart (const Comm::ConnectionPointer &client, StoreEntry *, HttpRequest *) |
Same as Start() but no master xaction info (AccessLogEntry) available. More... | |
static time_t | ForwardTimeout (const time_t fwdStart) |
time left to finish the whole forwarding process (which started at fwdStart) More... | |
static bool | EnoughTimeToReForward (const time_t fwdStart) |
static void | HandleStoreAbort (FwdState *) |
called by Store if the entry is no longer usable More... | |
Public Attributes | |
StoreEntry * | entry |
HttpRequest * | request |
AccessLogEntryPointer | al |
info for the future access.log entry More... | |
bool | subscribed = false |
whether noteDestination() and noteDestinationsEnd() calls are allowed More... | |
bool | connected_okay |
TCP link ever opened properly. This affects retry of POST,PUT,CONNECT,etc. More... | |
bool | dont_retry |
bool | forward_completed |
bool | destinationsFound |
at least one candidate path found More... | |
Private Types | |
enum | PconnRace { raceImpossible , racePossible , raceHappened } |
possible pconn race states More... | |
Private Member Functions | |
CBDATA_CHILD (FwdState) | |
FwdState (const Comm::ConnectionPointer &client, StoreEntry *, HttpRequest *, const AccessLogEntryPointer &alp) | |
void | start (Pointer aSelf) |
void | stopAndDestroy (const char *reason) |
ends forwarding; relies on refcounting so the effect may not be immediate More... | |
void | noteDestination (Comm::ConnectionPointer conn) override |
called when a new unique destination has been found More... | |
void | noteDestinationsEnd (ErrorState *selectionError) override |
bool | transporting () const |
void | noteConnection (HappyConnOpenerAnswer &) |
void | doneWithRetries () |
void | completed () |
void | retryOrBail () |
void | usePinned () |
send request on an existing connection dedicated to the requesting client More... | |
bool | pinnedCanRetry () const |
template<typename StepStart > | |
void | advanceDestination (const char *stepDescription, const Comm::ConnectionPointer &conn, const StepStart &startStep) |
starts a preparation step for an established connection; retries on failures More... | |
ErrorState * | makeConnectingError (const err_type type) const |
void | connectedToPeer (Security::EncryptorAnswer &answer) |
called when all negotiations with the TLS-speaking peer have been completed More... | |
void | establishTunnelThruProxy (const Comm::ConnectionPointer &) |
void | tunnelEstablishmentDone (Http::TunnelerAnswer &answer) |
resumes operations after the (possibly failed) HTTP CONNECT exchange More... | |
void | secureConnectionToPeerIfNeeded (const Comm::ConnectionPointer &) |
handles an established TCP connection to peer (including origin servers) More... | |
void | secureConnectionToPeer (const Comm::ConnectionPointer &) |
encrypts an established TCP connection to peer (including origin servers) More... | |
void | successfullyConnectedToPeer (const Comm::ConnectionPointer &) |
called when all negotiations with the peer have been completed More... | |
void | closeServerConnection (const char *reason) |
stops monitoring server connection for closure and updates pconn stats More... | |
void | syncWithServerConn (const Comm::ConnectionPointer &server, const char *host, const bool reused) |
commits to using the given open to-peer connection More... | |
void | syncHierNote (const Comm::ConnectionPointer &server, const char *host) |
bool | exhaustedTries () const |
whether we have used up all permitted forwarding attempts More... | |
void | updateAttempts (int) |
sets n_tries to the given value (while keeping ALE, if any, in sync) More... | |
time_t | connectingTimeout (const Comm::ConnectionPointer &conn) const |
void | cancelStep (const char *reason) |
void | notifyConnOpener () |
makes sure connection opener knows that the destinations have changed More... | |
void | reactToZeroSizeObject () |
ERR_ZERO_SIZE_OBJECT requires special adjustments. More... | |
void | updateAleWithFinalError () |
updates ALE when we finalize the transaction error (if any) More... | |
virtual void | finalizedInCbdataChild ()=0 |
hack: ensure CBDATA_CHILD() after a toCbdata()-defining CBDATA_INTERMEDIATE() More... | |
Static Private Member Functions | |
static void | logReplyStatus (int tries, const Http::StatusCode status) |
static void | RegisterWithCacheManager (void) |
Private Attributes | |
Pointer | self |
ErrorState * | err |
Comm::ConnectionPointer | clientConn |
a possibly open connection to the client. More... | |
time_t | start_t |
int | n_tries |
the number of forwarding attempts so far More... | |
struct { | |
bool connected_okay | |
TCP link ever opened properly. This affects retry of POST,PUT,CONNECT,etc. More... | |
bool dont_retry | |
bool forward_completed | |
bool destinationsFound | |
at least one candidate path found More... | |
} | flags |
JobWait< HappyConnOpener > | transportWait |
waits for a transport connection to the peer to be established/opened More... | |
JobWait< Security::PeerConnector > | encryptionWait |
waits for the established transport connection to be secured/encrypted More... | |
JobWait< Http::Tunneler > | peerWait |
bool | waitingForDispatched |
whether we are waiting for the last dispatch()ed activity to end More... | |
ResolvedPeersPointer | destinations |
paths for forwarding the request More... | |
Comm::ConnectionPointer | serverConn |
a successfully opened connection to a server. More... | |
PeerConnectionPointer | destinationReceipt |
peer selection result (or nil) More... | |
AsyncCall::Pointer | closeHandler |
The serverConn close handler. More... | |
PconnRace | pconnRace |
current pconn race state More... | |
const char * | storedWholeReply_ |
Detailed Description
Definition at line 52 of file FwdState.h.
Member Typedef Documentation
◆ Pointer
typedef RefCount<FwdState> FwdState::Pointer |
Definition at line 57 of file FwdState.h.
Member Enumeration Documentation
◆ PconnRace
|
private |
Enumerator | |
---|---|
raceImpossible | |
racePossible | |
raceHappened |
Definition at line 209 of file FwdState.h.
Constructor & Destructor Documentation
◆ ~FwdState()
|
override |
Definition at line 308 of file FwdState.cc.
References cancelStep(), closeServerConnection(), completed(), debugs, doneWithRetries(), entry, err, flags, HTTPMSGUNLOCK(), Comm::IsConnOpen(), request, serverConn, StoreEntry::unlock(), and StoreEntry::unregisterAbortCallback().
◆ FwdState()
|
private |
Definition at line 120 of file FwdState.cc.
References debugs, flags, HTTPMSGLOCK(), StoreEntry::lock(), request, and StoreEntry::url().
Referenced by Start().
Member Function Documentation
◆ advanceDestination()
|
private |
Definition at line 821 of file FwdState.cc.
References al, closePendingConnection(), conn, CurrentException(), debugs, err, ERR_GATEWAY_FAILURE, fail(), request, retryOrBail(), and Http::scInternalServerError.
Referenced by noteConnection(), and secureConnectionToPeerIfNeeded().
◆ cancelStep()
|
private |
Notify a pending subtask, if any, that we no longer need its help. We do not have to do this – the subtask job will eventually end – but ending it earlier reduces waste and may reduce DoS attack surface.
Definition at line 198 of file FwdState.cc.
References JobWaitBase::cancel(), encryptionWait, peerWait, and transportWait.
Referenced by ~FwdState(), and stopAndDestroy().
◆ CBDATA_CHILD()
|
private |
◆ checkRetriable()
bool FwdState::checkRetriable | ( | ) |
Definition at line 734 of file FwdState.cc.
References Http::Message::body_pipe, HttpRequestMethod::isHttpSafe(), HttpRequestMethod::isIdempotent(), HttpRequest::method, and request.
Referenced by checkRetry(), and connectStart().
◆ checkRetry()
bool FwdState::checkRetry | ( | ) |
Definition at line 690 of file FwdState.cc.
References HttpRequest::bodyNibbled(), checkRetriable(), debugs, EnoughTimeToReForward(), entry, exhaustedTries(), flags, HttpRequest::flags, StoreEntry::isEmpty(), RequestFlags::pinned, pinnedCanRetry(), request, self, shutting_down, start_t, STORE_PENDING, and StoreEntry::store_status.
Referenced by retryOrBail().
◆ closePendingConnection()
void FwdState::closePendingConnection | ( | const Comm::ConnectionPointer & | conn, |
const char * | reason | ||
) |
Definition at line 96 of file FwdState.cc.
References assert, closeHandler, conn, debugs, fd_table, fwdPconnPool, Comm::IsConnOpen(), PconnPool::noteUses(), and serverConn.
Referenced by advanceDestination(), connectedToPeer(), noteConnection(), and tunnelEstablishmentDone().
◆ closeServerConnection()
|
private |
Definition at line 108 of file FwdState.cc.
References assert, Comm::Connection::close(), closeHandler, comm_remove_close_handler(), debugs, Comm::Connection::fd, fd_table, fwdPconnPool, Comm::IsConnOpen(), PconnPool::noteUses(), and serverConn.
Referenced by ~FwdState(), and HandleStoreAbort().
◆ complete()
void FwdState::complete | ( | ) |
FooClient modules call fwdComplete() when they are done downloading an object. Then, we either 1) re-forward the request somewhere else if needed, or 2) call storeComplete() to finish it off
Definition at line 531 of file FwdState.cc.
References MemObject::baseReply(), completed(), debugs, destinationReceipt, entry, Comm::IsConnOpen(), logReplyStatus(), StoreEntry::mem(), StoreEntry::mem_obj, n_tries, reforward(), StoreEntry::reset(), serverConn, serverConnection(), HttpReply::sline, Http::StatusLine::status(), stopAndDestroy(), storedWholeReply_, unregister(), StoreEntry::url(), useDestinations(), and waitingForDispatched.
Referenced by Client::completeForwarding(), connectedToPeer(), dispatch(), and WhoisState::readReply().
◆ completed()
|
private |
Definition at line 257 of file FwdState.cc.
References al, assert, CallJobHere1, HttpRequest::clientConnectionManager, StoreEntry::completeSuccessfully(), StoreEntry::completeTruncated(), DBG_IMPORTANT, debugs, EBIT_TEST, entry, ENTRY_ABORTED, ENTRY_FWD_HDR_WAIT, err, ERR_READ_ERROR, errorAppendEntry(), fail(), flags, HttpRequest::flags, StoreEntry::flags, HttpRequest::hier, ConnStateData::httpsPeeked(), StoreEntry::isEmpty(), StoreEntry::mem_obj, request, Http::scBadGateway, RequestFlags::sslPeek, HierarchyLogEntry::stopPeerClock(), STORE_PENDING, StoreEntry::store_status, storedWholeReply_, storePendingNClients(), updateAleWithFinalError(), and CbcPointer< Cbc >::valid().
Referenced by ~FwdState(), and complete().
◆ connectDone()
void FwdState::connectDone | ( | const Comm::ConnectionPointer & | conn, |
Comm::Flag | status, | ||
int | xerrno | ||
) |
◆ connectedToPeer()
|
private |
Definition at line 1015 of file FwdState.cc.
References StoreEntry::abort(), al, assert, CbcPointer< Cbc >::clear(), closePendingConnection(), complete(), Security::EncryptorAnswer::conn, encryptionWait, entry, ERR_CANNOT_FORWARD, error(), Security::EncryptorAnswer::error, fail(), Comm::Connection::fd, fd_table, JobWaitBase::finish(), flags, CbcPointer< Cbc >::get(), Comm::IsConnOpen(), request, retryOrBail(), Http::scServiceUnavailable, successfullyConnectedToPeer(), and Security::EncryptorAnswer::tunneled.
Referenced by secureConnectionToPeer().
◆ connectingTimeout()
|
private |
- Returns
- the time left for this connection to become connected or 1 second if it is less than one second left
Definition at line 1439 of file FwdState.cc.
References conn, positiveTimeout(), and start_t.
Referenced by establishTunnelThruProxy(), and secureConnectionToPeer().
◆ connectStart()
void FwdState::connectStart | ( | ) |
Called after forwarding path selection (via peer select) has taken place and whenever forwarding needs to attempt a new connection (routing failover). We have a vector of possible localIP->remoteIP paths now ready to start being connected.
Definition at line 1116 of file FwdState.cc.
References SquidConfig::accessList, ACLFilledChecklist::al, al, Acl::Answer::allowed(), assert, asyncCallback, checkRetriable(), HttpRequest::clearError(), Config, debugs, destinations, ResolvedPeers::empty(), entry, err, ACLChecklist::fastCheck(), HttpRequest::hier, AnyP::Uri::host(), Must, n_tries, noteConnection(), ResolvedPeers::notificationPending, pconnRace, HttpRequest::pinnedConnection(), raceHappened, request, SquidConfig::serverPconnForNonretriable, JobWait< Job >::start(), start_t, HierarchyLogEntry::startPeerClock(), ACLFilledChecklist::syncAle(), transporting(), transportWait, HttpRequest::url, and StoreEntry::url().
Referenced by useDestinations().
◆ dispatch()
void FwdState::dispatch | ( | ) |
Definition at line 1189 of file FwdState.cc.
References StoreEntry::abort(), al, assert, CallJobHere1, clientConn, HttpRequest::clientConnectionManager, Comm::Connection::close(), complete(), DBG_IMPORTANT, debugs, Ip::Qos::dirOpened, EBIT_SET, entry, ENTRY_DISPATCHED, ERR_UNSUP_REQ, fail(), fatal_dump(), Comm::Connection::fd, fd_note(), fd_table, flags, HttpRequest::flags, StoreEntry::flags, RequestFlags::ftpNative, Ip::Qos::getNfConnmark(), AnyP::Uri::getScheme(), Ip::Qos::getTosFromServer(), AnyP::Uri::host(), ConnStateData::httpsPeeked(), httpStart(), Comm::IsConnOpen(), StoreEntry::locked(), HttpRequest::method, netdbPingSite(), fde::nfConnmarkFromServer, StoreEntry::ping_status, PING_WAITING, HttpRequest::prepForDirect(), HttpRequest::prepForPeering(), AnyP::PROTO_FTP, AnyP::PROTO_HTTP, AnyP::PROTO_HTTPS, AnyP::PROTO_URN, AnyP::PROTO_WAIS, AnyP::PROTO_WHOIS, request, Http::scBadRequest, serverConn, serverConnection(), RequestFlags::sslPeek, Ftp::StartGateway(), Ftp::StartRelay(), Ip::Qos::TheConfig, unregister(), HttpRequest::url, StoreEntry::url(), waitingForDispatched, and whoisStart().
Referenced by noteConnection(), successfullyConnectedToPeer(), and usePinned().
◆ doneWithRetries()
|
private |
Definition at line 797 of file FwdState.cc.
References Http::Message::body_pipe, BodyPipe::expectNoConsumption(), and request.
Referenced by ~FwdState(), and retryOrBail().
◆ dontRetry() [1/2]
|
inline |
Definition at line 96 of file FwdState.h.
References flags.
Referenced by HttpStateData::continueAfterParsingHeader(), Client::handleAdaptationBlocked(), Client::handledEarlyAdaptationAbort(), Client::handleRequestBodyProducerAborted(), and Client::sendBodyIsTooLargeError().
◆ dontRetry() [2/2]
|
inline |
Definition at line 98 of file FwdState.h.
References flags.
◆ EnoughTimeToReForward()
|
static |
Whether there is still time to re-try after a previous connection failure.
- Parameters
-
fwdStart The start time of the peer selection/connection process.
Definition at line 436 of file FwdState.cc.
References ForwardTimeout(), and fwdStart().
Referenced by checkRetry(), and TunnelStateData::checkRetry().
◆ establishTunnelThruProxy()
|
private |
Definition at line 909 of file FwdState.cc.
References al, asyncCallback, conn, connectingTimeout(), entry, StoreEntry::mem_obj, MemObject::mostBytesAllowed(), Must, peerWait, request, JobWait< Job >::start(), and tunnelEstablishmentDone().
◆ exhaustedTries()
|
private |
Definition at line 1411 of file FwdState.cc.
References Config, SquidConfig::forward_max_tries, and n_tries.
Referenced by checkRetry(), and reforward().
◆ fail()
void FwdState::fail | ( | ErrorState * | err | ) |
Definition at line 463 of file FwdState.cc.
References debugs, destinationReceipt, entry, err, err_type_str, ERR_ZERO_SIZE_OBJECT, ErrorState::httpStatus, reactToZeroSizeObject(), ErrorState::request, request, Http::StatusCodeString(), ErrorState::type, and StoreEntry::url().
Referenced by advanceDestination(), completed(), connectedToPeer(), HttpStateData::continueAfterParsingHeader(), dispatch(), HttpStateData::drop1xx(), Client::handleAdaptationBlocked(), Client::handledEarlyAdaptationAbort(), HttpStateData::handleRequestBodyProducerAborted(), HttpStateData::httpTimeout(), HttpStateData::markPrematureReplyBodyEofFailure(), noteConnection(), noteDestinationsEnd(), WhoisState::readReply(), HttpStateData::readReply(), Client::sendBodyIsTooLargeError(), Client::sentRequestBody(), tunnelEstablishmentDone(), useDestinations(), usePinned(), and HttpStateData::wroteLast().
◆ finalizedInCbdataChild()
|
privatepure virtualinherited |
◆ ForwardTimeout()
|
static |
Definition at line 428 of file FwdState.cc.
References Config, diffOrZero(), SquidConfig::forward, fwdStart(), squid_curtime, and SquidConfig::Timeout.
Referenced by Comm::Connection::connectTimeout(), EnoughTimeToReForward(), and HappyConnOpener::ranOutOfTimeOrAttempts().
◆ fwdStart()
|
static |
Definition at line 412 of file FwdState.cc.
References clientConn, entry, request, and Start().
Referenced by asnCacheStart(), EnoughTimeToReForward(), ForwardTimeout(), netdbExchangeStart(), and peerDigestRequest().
◆ HandleStoreAbort()
|
static |
Definition at line 83 of file FwdState.cc.
References closeServerConnection(), debugs, Comm::IsConnOpen(), serverConnection(), and stopAndDestroy().
Referenced by start().
◆ handleUnregisteredServerEnd()
void FwdState::handleUnregisteredServerEnd | ( | ) |
Definition at line 805 of file FwdState.cc.
References assert, debugs, destinationReceipt, entry, err, Comm::IsConnOpen(), retryOrBail(), self, serverConn, StoreEntry::url(), and waitingForDispatched.
Referenced by Client::swanSong().
◆ initModule()
|
static |
Definition at line 1385 of file FwdState.cc.
References RegisterWithCacheManager().
Referenced by mainInitialize().
◆ logReplyStatus()
|
staticprivate |
Definition at line 1397 of file FwdState.cc.
References assert, FwdReplyCodes, MAX_FWD_STATS_IDX, and Http::scInvalidHeader.
Referenced by complete().
◆ makeConnectingError()
|
private |
◆ markStoredReplyAsWhole()
void FwdState::markStoredReplyAsWhole | ( | const char * | whyWeAreSure | ) |
Mark reply as written to Store in its entirety, including the header and any body. If the reply has a body, the entire body has to be stored.
Definition at line 580 of file FwdState.cc.
References debugs, EBIT_TEST, entry, ENTRY_ABORTED, StoreEntry::flags, and storedWholeReply_.
Referenced by Client::endAdaptedBodyConsumption(), Client::handleAdaptedHeader(), Client::markParsedVirginReplyAsWhole(), and WhoisState::readReply().
◆ noteConnection()
|
private |
called when a to-peer connection has been successfully obtained or when all candidate destinations have been tried and all have failed
Definition at line 841 of file FwdState.cc.
References advanceDestination(), al, assert, CbcPointer< Cbc >::clear(), closePendingConnection(), HappyConnOpenerAnswer::conn, destinationReceipt, dispatch(), ERR_CANNOT_FORWARD, error(), HappyConnOpenerAnswer::error, fail(), Comm::Connection::fd, fd_table, JobWaitBase::finish(), flags, HttpRequest::flags, CbcPointer< Cbc >::get(), Comm::Connection::getPeer(), AnyP::Uri::host(), Comm::IsConnOpen(), HttpRequest::method, Http::METHOD_CONNECT, Must, HappyConnOpenerAnswer::n_tries, request, retryOrBail(), HappyConnOpenerAnswer::reused, Http::scServiceUnavailable, secureConnectionToPeerIfNeeded(), RequestFlags::sslBumped, RequestFlags::sslPeek, syncHierNote(), syncWithServerConn(), transportWait, updateAttempts(), and HttpRequest::url.
Referenced by connectStart().
◆ noteDestination()
|
overrideprivatevirtual |
Implements PeerSelectionInitiator.
Definition at line 592 of file FwdState.cc.
References ResolvedPeers::addPath(), assert, debugs, destinations, ResolvedPeers::empty(), flags, notifyConnOpener(), transporting(), transportWait, useDestinations(), and usePinned().
◆ noteDestinationsEnd()
|
overrideprivatevirtual |
called when there will be no more noteDestination() calls
- Parameters
-
error is a possible reason why no destinations were found; it is guaranteed to be nil if there was at least one noteDestination() call
Implements PeerSelectionInitiator.
Definition at line 621 of file FwdState.cc.
References assert, debugs, destinations, ResolvedPeers::destinationsFinalized, err, fail(), flags, Must, notifyConnOpener(), stopAndDestroy(), PeerSelectionInitiator::subscribed, transporting(), and transportWait.
◆ notifyConnOpener()
|
private |
Definition at line 660 of file FwdState.cc.
References CallJobHere, debugs, destinations, JobWait< Job >::job(), ResolvedPeers::notificationPending, and transportWait.
Referenced by noteDestination(), and noteDestinationsEnd().
◆ pconnPush()
void FwdState::pconnPush | ( | Comm::ConnectionPointer & | conn, |
const char * | domain | ||
) |
◆ pinnedCanRetry()
|
private |
whether a pinned to-peer connection can be replaced with another one (in order to retry or reforward a failed request)
Definition at line 1417 of file FwdState.cc.
References assert, HttpRequest::flags, pconnRace, RequestFlags::pinned, raceHappened, request, and RequestFlags::sslBumped.
Referenced by checkRetry(), and reforward().
◆ reactToZeroSizeObject()
|
private |
Definition at line 481 of file FwdState.cc.
References assert, debugs, destinationReceipt, destinations, err, ERR_ZERO_SIZE_OBJECT, pconnRace, HttpRequest::pinnedConnection(), raceHappened, racePossible, ResolvedPeers::reinstatePath(), request, and ErrorState::type.
Referenced by fail().
◆ reforward()
int FwdState::reforward | ( | ) |
Definition at line 1311 of file FwdState.cc.
References assert, MemObject::baseReply(), HttpRequest::bodyNibbled(), debugs, destinations, EBIT_TEST, ResolvedPeers::empty(), entry, ENTRY_ABORTED, ENTRY_FWD_HDR_WAIT, exhaustedTries(), HttpRequest::flags, StoreEntry::flags, Http::IsReforwardableStatus(), StoreEntry::mem(), StoreEntry::mem_obj, RequestFlags::pinned, pinnedCanRetry(), request, HttpReply::sline, Http::StatusLine::status(), STORE_PENDING, StoreEntry::store_status, PeerSelectionInitiator::subscribed, and StoreEntry::url().
Referenced by complete().
◆ RegisterWithCacheManager()
|
staticprivate |
Definition at line 1391 of file FwdState.cc.
References fwdStats, and Mgr::RegisterAction().
Referenced by initModule().
◆ retryOrBail()
|
private |
Definition at line 772 of file FwdState.cc.
References al, checkRetry(), debugs, doneWithRetries(), entry, err, ERR_SHUTTING_DOWN, errorAppendEntry(), HttpRequest::hier, StoreEntry::isEmpty(), n_tries, request, Http::scServiceUnavailable, self, shutting_down, squid_curtime, start_t, stopAndDestroy(), HierarchyLogEntry::stopPeerClock(), and useDestinations().
Referenced by advanceDestination(), connectedToPeer(), handleUnregisteredServerEnd(), noteConnection(), serverClosed(), and tunnelEstablishmentDone().
◆ secureConnectionToPeer()
|
private |
Definition at line 997 of file FwdState.cc.
References al, asyncCallback, clientConn, conn, connectedToPeer(), connectingTimeout(), encryptionWait, HttpRequest::flags, Security::PeerConnector::noteFwdPconnUse, request, RequestFlags::sslPeek, and JobWait< Job >::start().
Referenced by secureConnectionToPeerIfNeeded().
◆ secureConnectionToPeerIfNeeded()
|
private |
Definition at line 968 of file FwdState.cc.
References advanceDestination(), assert, conn, HttpRequest::flags, AnyP::Uri::getScheme(), HttpRequest::method, Http::METHOD_CONNECT, RequestFlags::pinned, AnyP::PROTO_HTTPS, request, secureConnectionToPeer(), RequestFlags::sslBumped, RequestFlags::sslPeek, successfullyConnectedToPeer(), and HttpRequest::url.
Referenced by noteConnection(), and tunnelEstablishmentDone().
◆ serverClosed()
void FwdState::serverClosed | ( | ) |
Definition at line 747 of file FwdState.cc.
References closeHandler, debugs, destinationReceipt, Comm::Connection::fd, fd_table, fwdPconnPool, Comm::IsConnOpen(), Comm::Connection::noteClosure(), PconnPool::noteUses(), retryOrBail(), serverConn, and waitingForDispatched.
Referenced by fwdServerClosedWrapper().
◆ serverConnection()
|
inline |
return a ConnectionPointer to the current server connection (may or may not be open)
Definition at line 104 of file FwdState.h.
References serverConn.
Referenced by Ftp::Client::Client(), HttpStateData::HttpStateData(), complete(), dispatch(), HandleStoreAbort(), successfullyConnectedToPeer(), unregister(), and whoisStart().
◆ Start()
|
static |
This is the entry point for client-side to start forwarding a transaction. It is a static method that may or may not allocate a FwdState.
- Note
- client_addr == no_addr indicates this is an "internal" request from peer_digest.c, asn.c, netdb.c, etc and should always be allowed. yuck, I know.
Check if this host is allowed to fetch MISSES from us (miss_access). Intentionally replace the src_addr automatically selected by the checklist code we do NOT want the indirect client address to be tested here.
Definition at line 341 of file FwdState.cc.
References FwdState(), SquidConfig::accessList, aclGetDenyInfoPage(), AclMatchedName, ACLFilledChecklist::al, al, HttpRequest::client_addr, clientConn, Config, debugs, Acl::Answer::denied(), SquidConfig::denyInfoList, entry, ERR_FORWARDING_DENIED, ERR_NONE, ERR_SHUTTING_DOWN, errorAppendEntry(), ACLChecklist::fastCheck(), HttpRequest::flags, AnyP::Uri::getScheme(), RequestFlags::internal, internalStart(), Ip::Address::isNoAddr(), StoreEntry::mem_obj, SquidConfig::miss, AnyP::PROTO_URN, request, MemObject::request, Http::scForbidden, Http::scServiceUnavailable, shutting_down, ACLFilledChecklist::src_addr, start(), ACLFilledChecklist::syncAle(), HttpRequest::url, StoreEntry::url(), and urnStart().
Referenced by fwdStart(), ConnStateData::parseTlsHandshake(), clientReplyContext::processExpired(), clientReplyContext::processMiss(), UrnState::start(), and ConnStateData::startPeekAndSplice().
◆ start()
|
private |
Definition at line 144 of file FwdState.cc.
References al, asyncCall(), cbdataDialer(), SquidConfig::client_dst_passthru, Config, entry, HttpRequest::flags, RequestFlags::ftpNative, HandleStoreAbort(), RequestFlags::hostVerified, RequestFlags::intercepted, RequestFlags::interceptTproxy, SquidConfig::onoff, RequestFlags::pinned, RequestFlags::redirected, StoreEntry::registerAbortCallback(), request, self, and PeerSelectionInitiator::startSelectingDestinations().
Referenced by Start().
◆ startSelectingDestinations()
|
inherited |
Initiates asynchronous peer selection that eventually results in zero or more noteDestination() calls and exactly one noteDestinationsEnd() call.
Definition at line 335 of file peer_select.cc.
References peerSelect(), and PeerSelectionInitiator::subscribed.
Referenced by start(), and tunnelStart().
◆ stopAndDestroy()
|
private |
Definition at line 183 of file FwdState.cc.
References cancelStep(), debugs, self, and PeerSelectionInitiator::subscribed.
Referenced by complete(), HandleStoreAbort(), noteDestinationsEnd(), retryOrBail(), useDestinations(), and usePinned().
◆ successfullyConnectedToPeer()
|
private |
Definition at line 1051 of file FwdState.cc.
References CallJobHere1, HttpRequest::clientConnectionManager, conn, dispatch(), AnyP::Uri::host(), NoteOutgoingConnectionSuccess(), ConnStateData::notePeerConnection(), request, serverConnection(), syncWithServerConn(), and HttpRequest::url.
Referenced by connectedToPeer(), and secureConnectionToPeerIfNeeded().
◆ syncHierNote()
|
private |
Definition at line 1086 of file FwdState.cc.
References al, AccessLogEntry::hier, HttpRequest::hier, request, HierarchyLogEntry::resetPeerNotes(), and server.
Referenced by noteConnection(), syncWithServerConn(), and usePinned().
◆ syncWithServerConn()
|
private |
Definition at line 1066 of file FwdState.cc.
References closeHandler, comm_add_close_handler(), conn, Comm::Connection::fd, fwdServerClosedWrapper, Comm::IsConnOpen(), Must, pconnRace, raceImpossible, racePossible, request, ResetMarkingsToServer(), serverConn, and syncHierNote().
Referenced by noteConnection(), successfullyConnectedToPeer(), and usePinned().
◆ toCbdata()
|
pure virtualinherited |
Referenced by AsyncJob::callException(), and AsyncJob::callStart().
◆ transporting()
|
private |
Whether a forwarding attempt to some selected destination X is in progress (after successfully opening/reusing a transport connection to X). See also: transportWait
Definition at line 574 of file FwdState.cc.
References encryptionWait, peerWait, and waitingForDispatched.
Referenced by connectStart(), noteDestination(), and noteDestinationsEnd().
◆ tunnelEstablishmentDone()
|
private |
Definition at line 930 of file FwdState.cc.
References al, CbcPointer< Cbc >::clear(), closePendingConnection(), Http::TunnelerAnswer::conn, DBG_IMPORTANT, debugs, ERR_CANNOT_FORWARD, ERR_CONNECT_FAIL, error(), fail(), Comm::Connection::fd, fd_table, JobWaitBase::finish(), CbcPointer< Cbc >::get(), Comm::IsConnOpen(), SBuf::isEmpty(), Http::TunnelerAnswer::leftovers, SBuf::length(), Must, peerWait, Http::TunnelerAnswer::positive(), request, retryOrBail(), Http::scBadGateway, Http::scServiceUnavailable, secureConnectionToPeerIfNeeded(), and Http::TunnelerAnswer::squidError.
Referenced by establishTunnelThruProxy().
◆ unregister() [1/2]
void FwdState::unregister | ( | Comm::ConnectionPointer & | conn | ) |
Frees fwdState without closing FD or generating an abort
Definition at line 504 of file FwdState.cc.
References assert, closeHandler, comm_remove_close_handler(), conn, debugs, destinationReceipt, entry, Comm::IsConnOpen(), serverConn, serverConnection(), and StoreEntry::url().
Referenced by HttpStateData::closeServer(), complete(), dispatch(), HttpStateData::proceedAfter1xx(), HttpStateData::processReplyBody(), and unregister().
◆ unregister() [2/2]
void FwdState::unregister | ( | int | fd | ) |
Definition at line 517 of file FwdState.cc.
References assert, debugs, entry, serverConn, serverConnection(), unregister(), and StoreEntry::url().
◆ updateAleWithFinalError()
|
private |
Definition at line 238 of file FwdState.cc.
References LogTagsErrors::aborted, al, AccessLogEntry::cache, AccessLogEntry::CacheDetails::code, ErrorState::detail, ErrorState::detailError(), err, LogTags::err, ERR_NONE, ERR_READ_TIMEOUT, Helper::Error, MakeNamedErrorDetail(), LogTagsErrors::timedout, ErrorState::type, LogTagsErrors::update(), AccessLogEntry::updateError(), and ErrorState::xerrno.
Referenced by completed().
◆ updateAttempts()
|
private |
Definition at line 1096 of file FwdState.cc.
References al, Assure, debugs, n_tries, and AccessLogEntry::requestAttempts.
Referenced by noteConnection(), and usePinned().
◆ useDestinations()
void FwdState::useDestinations | ( | ) |
This is the real beginning of server connection. Call it whenever the forwarding server destination has changed and a new one needs to be opened. Produces the cannot-forward error on fail if no better error exists.
Definition at line 442 of file FwdState.cc.
References al, connectStart(), debugs, destinations, ResolvedPeers::empty(), entry, err, ERR_CANNOT_FORWARD, fail(), request, Http::scInternalServerError, stopAndDestroy(), PeerSelectionInitiator::subscribed, and StoreEntry::url().
Referenced by complete(), noteDestination(), and retryOrBail().
◆ usePinned()
|
private |
Definition at line 1152 of file FwdState.cc.
References al, assert, RequestFlags::auth, ConnStateData::BorrowPinnedConnection(), debugs, dispatch(), fail(), HttpRequest::flags, AnyP::Uri::host(), n_tries, RequestFlags::pinned, HttpRequest::pinnedConnection(), request, serverConn, stopAndDestroy(), syncHierNote(), syncWithServerConn(), updateAttempts(), and HttpRequest::url.
Referenced by noteDestination().
Member Data Documentation
◆ al
AccessLogEntryPointer FwdState::al |
Definition at line 170 of file FwdState.h.
Referenced by Client::adaptOrFinalizeReply(), advanceDestination(), Client::blockCaching(), HttpStateData::buildRequestPrefix(), completed(), connectedToPeer(), connectStart(), HttpStateData::continueAfterParsingHeader(), dispatch(), HttpStateData::drop1xx(), establishTunnelThruProxy(), HttpStateData::finishingBrokenPost(), HttpStateData::forwardUpgrade(), ftpFail(), ftpSendReply(), HttpStateData::handle1xx(), Client::handleAdaptationBlocked(), Client::handledEarlyAdaptationAbort(), HttpStateData::handleRequestBodyProducerAborted(), HttpStateData::httpTimeout(), HttpStateData::markPrematureReplyBodyEofFailure(), noteConnection(), WhoisState::readReply(), HttpStateData::readReply(), retryOrBail(), secureConnectionToPeer(), Client::sendBodyIsTooLargeError(), Client::sentRequestBody(), Client::setFinalReply(), Client::setVirginReply(), Start(), start(), Client::startAdaptation(), syncHierNote(), tunnelEstablishmentDone(), updateAleWithFinalError(), updateAttempts(), useDestinations(), usePinned(), and HttpStateData::wroteLast().
◆ clientConn
|
private |
Definition at line 178 of file FwdState.h.
Referenced by dispatch(), fwdStart(), secureConnectionToPeer(), and Start().
◆ closeHandler
|
private |
Definition at line 206 of file FwdState.h.
Referenced by closePendingConnection(), closeServerConnection(), serverClosed(), syncWithServerConn(), and unregister().
◆ connected_okay
bool FwdState::connected_okay |
Definition at line 183 of file FwdState.h.
◆ destinationReceipt
|
private |
Definition at line 204 of file FwdState.h.
Referenced by complete(), fail(), handleUnregisteredServerEnd(), noteConnection(), reactToZeroSizeObject(), serverClosed(), and unregister().
◆ destinations
|
private |
Definition at line 202 of file FwdState.h.
Referenced by connectStart(), noteDestination(), noteDestinationsEnd(), notifyConnOpener(), reactToZeroSizeObject(), reforward(), and useDestinations().
◆ destinationsFound
bool FwdState::destinationsFound |
Definition at line 186 of file FwdState.h.
◆ dont_retry
bool FwdState::dont_retry |
Definition at line 184 of file FwdState.h.
◆ encryptionWait
|
private |
Definition at line 193 of file FwdState.h.
Referenced by cancelStep(), connectedToPeer(), secureConnectionToPeer(), and transporting().
◆ entry
StoreEntry* FwdState::entry |
Definition at line 168 of file FwdState.h.
Referenced by Client::Client(), ~FwdState(), checkRetry(), complete(), completed(), connectedToPeer(), connectStart(), dispatch(), establishTunnelThruProxy(), fail(), fwdStart(), handleUnregisteredServerEnd(), httpStart(), markStoredReplyAsWhole(), reforward(), retryOrBail(), Start(), start(), unregister(), useDestinations(), and whoisStart().
◆ err
|
private |
Definition at line 177 of file FwdState.h.
Referenced by ~FwdState(), advanceDestination(), completed(), connectStart(), fail(), handleUnregisteredServerEnd(), noteDestinationsEnd(), reactToZeroSizeObject(), retryOrBail(), updateAleWithFinalError(), and useDestinations().
◆
struct { ... } FwdState::flags |
Referenced by FwdState(), ~FwdState(), checkRetry(), completed(), connectedToPeer(), dispatch(), dontRetry(), noteConnection(), noteDestination(), and noteDestinationsEnd().
◆ forward_completed
bool FwdState::forward_completed |
Definition at line 185 of file FwdState.h.
◆ n_tries
|
private |
Definition at line 180 of file FwdState.h.
Referenced by complete(), connectStart(), exhaustedTries(), retryOrBail(), updateAttempts(), and usePinned().
◆ pconnRace
|
private |
Definition at line 210 of file FwdState.h.
Referenced by connectStart(), pinnedCanRetry(), reactToZeroSizeObject(), and syncWithServerConn().
◆ peerWait
|
private |
waits for an HTTP CONNECT tunnel through a cache_peer to be negotiated over the (encrypted, if needed) transport connection to that cache_peer
Definition at line 197 of file FwdState.h.
Referenced by cancelStep(), establishTunnelThruProxy(), transporting(), and tunnelEstablishmentDone().
◆ request
HttpRequest* FwdState::request |
Definition at line 169 of file FwdState.h.
Referenced by FwdState(), ~FwdState(), advanceDestination(), checkRetriable(), checkRetry(), completed(), connectedToPeer(), connectStart(), HttpStateData::continueAfterParsingHeader(), dispatch(), doneWithRetries(), establishTunnelThruProxy(), fail(), ftpFail(), fwdStart(), HttpStateData::handleRequestBodyProducerAborted(), httpStart(), HttpStateData::httpTimeout(), HttpStateData::markPrematureReplyBodyEofFailure(), noteConnection(), pinnedCanRetry(), reactToZeroSizeObject(), WhoisState::readReply(), HttpStateData::readReply(), reforward(), retryOrBail(), secureConnectionToPeer(), secureConnectionToPeerIfNeeded(), Client::sentRequestBody(), Start(), start(), successfullyConnectedToPeer(), syncHierNote(), syncWithServerConn(), tunnelEstablishmentDone(), useDestinations(), usePinned(), whoisStart(), and HttpStateData::wroteLast().
◆ self
|
private |
Definition at line 176 of file FwdState.h.
Referenced by checkRetry(), handleUnregisteredServerEnd(), retryOrBail(), start(), and stopAndDestroy().
◆ serverConn
|
private |
Definition at line 203 of file FwdState.h.
Referenced by ~FwdState(), closePendingConnection(), closeServerConnection(), complete(), dispatch(), handleUnregisteredServerEnd(), serverClosed(), serverConnection(), syncWithServerConn(), unregister(), and usePinned().
◆ start_t
|
private |
Definition at line 179 of file FwdState.h.
Referenced by checkRetry(), connectingTimeout(), connectStart(), and retryOrBail().
◆ storedWholeReply_
|
private |
Whether the entire reply (including any body) was written to Store. The string literal value is only used for debugging.
Definition at line 214 of file FwdState.h.
Referenced by complete(), completed(), and markStoredReplyAsWhole().
◆ subscribed
|
inherited |
Definition at line 46 of file PeerSelectState.h.
Referenced by TunnelStateData::commitToServer(), noteDestinationsEnd(), TunnelStateData::noteDestinationsEnd(), reforward(), TunnelStateData::retryOrBail(), TunnelStateData::sendError(), PeerSelectionInitiator::startSelectingDestinations(), stopAndDestroy(), and useDestinations().
◆ transportWait
|
private |
Definition at line 190 of file FwdState.h.
Referenced by cancelStep(), connectStart(), noteConnection(), noteDestination(), noteDestinationsEnd(), and notifyConnOpener().
◆ waitingForDispatched
|
private |
Definition at line 200 of file FwdState.h.
Referenced by complete(), dispatch(), handleUnregisteredServerEnd(), serverClosed(), and transporting().
The documentation for this class was generated from the following files:
- src/FwdState.h
- src/FwdState.cc