#include <pconn.h>
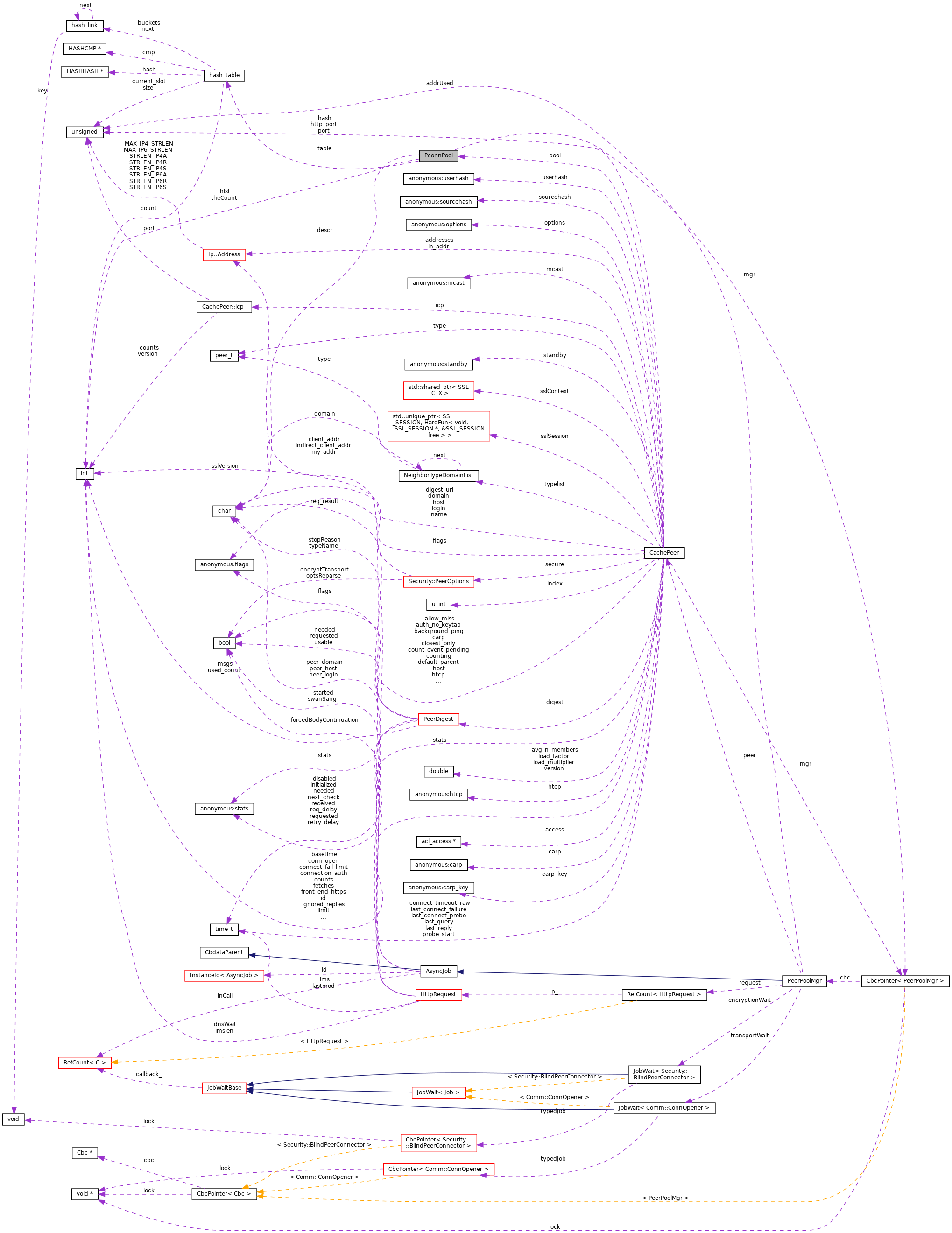
Public Member Functions | |
PconnPool (const char *aDescription, const CbcPointer< PeerPoolMgr > &aMgr) | |
~PconnPool () | |
void | moduleInit () |
void | push (const Comm::ConnectionPointer &serverConn, const char *domain) |
Comm::ConnectionPointer | pop (const Comm::ConnectionPointer &dest, const char *domain, bool keepOpen) |
void | count (int uses) |
void | dumpHist (StoreEntry *e) const |
void | dumpHash (StoreEntry *e) const |
void | unlinkList (IdleConnList *list) |
void | noteUses (int uses) |
void | closeN (int n) |
closes any n connections, regardless of their destination More... | |
int | count () const |
void | noteConnectionAdded () |
void | noteConnectionRemoved () |
void | notifyManager (const char *reason) |
Private Member Functions | |
Comm::ConnectionPointer | popStored (const Comm::ConnectionPointer &dest, const char *domain, const bool keepOpen) |
Static Private Member Functions | |
static const char * | key (const Comm::ConnectionPointer &destLink, const char *domain) |
Private Attributes | |
int | hist [PCONN_HIST_SZ] |
hash_table * | table |
const char * | descr |
CbcPointer< PeerPoolMgr > | mgr |
optional pool manager (for notifications) More... | |
int | theCount |
the number of pooled connections More... | |
Detailed Description
Manages idle persistent connections to a caller-defined set of servers (e.g., all HTTP servers). Uses a collection of IdleConnLists internally to list the individual open connections to each server. Controls lists existence and limits the total number of idle connections across the collection.
Constructor & Destructor Documentation
◆ PconnPool()
PconnPool::PconnPool | ( | const char * | aDescription, |
const CbcPointer< PeerPoolMgr > & | aMgr | ||
) |
Definition at line 383 of file pconn.cc.
References PconnModule::add(), PconnModule::GetInstance(), hash_create(), hash_string, hist, PCONN_HIST_SZ, and table.
◆ ~PconnPool()
PconnPool::~PconnPool | ( | ) |
Definition at line 403 of file pconn.cc.
References DeleteIdleConnList(), descr, PconnModule::GetInstance(), hashFreeItems(), hashFreeMemory(), PconnModule::remove(), and table.
Member Function Documentation
◆ closeN()
void PconnPool::closeN | ( | int | n | ) |
Definition at line 507 of file pconn.cc.
References closeN(), count(), hash_first(), hash_next(), Must, and table.
Referenced by closeN(), and PeerPoolMgr::closeOldConnections().
◆ count() [1/2]
|
inline |
◆ count() [2/2]
void PconnPool::count | ( | int | uses | ) |
Referenced by PeerPoolMgr::checkpoint(), and peerHasConnAvailable().
◆ dumpHash()
void PconnPool::dumpHash | ( | StoreEntry * | e | ) | const |
Definition at line 369 of file pconn.cc.
References hash_first(), hash_next(), storeAppendPrintf(), and table.
◆ dumpHist()
void PconnPool::dumpHist | ( | StoreEntry * | e | ) | const |
Definition at line 351 of file pconn.cc.
References descr, hist, PCONN_HIST_SZ, and storeAppendPrintf().
◆ key()
|
staticprivate |
Definition at line 331 of file pconn.cc.
References debugs, Comm::Connection::getPeer(), LOCAL_ARRAY, Comm::Connection::remote, SQUIDHOSTNAMELEN, and Ip::Address::toUrl().
Referenced by popStored(), and push().
◆ moduleInit()
void PconnPool::moduleInit | ( | ) |
◆ noteConnectionAdded()
|
inline |
◆ noteConnectionRemoved()
|
inline |
Definition at line 144 of file pconn.h.
References assert, and theCount.
Referenced by IdleConnList::closeN(), and IdleConnList::removeAt().
◆ noteUses()
void PconnPool::noteUses | ( | int | uses | ) |
Definition at line 536 of file pconn.cc.
References hist, and PCONN_HIST_SZ.
Referenced by FwdState::closePendingConnection(), FwdState::closeServerConnection(), Http::Tunneler::countFailingConnection(), Security::PeerConnector::countFailingConnection(), and FwdState::serverClosed().
◆ notifyManager()
void PconnPool::notifyManager | ( | const char * | reason | ) |
Definition at line 500 of file pconn.cc.
References PeerPoolMgr::Checkpoint(), mgr, and CbcPointer< Cbc >::valid().
Referenced by IdleConnList::findAndClose(), popStored(), and push().
◆ pop()
Comm::ConnectionPointer PconnPool::pop | ( | const Comm::ConnectionPointer & | dest, |
const char * | domain, | ||
bool | keepOpen | ||
) |
Returns either a pointer to a popped connection to dest or nil. Closes the connection before returning its pointer unless keepOpen. For connection going to a cache_peer, supports standby connection pools.
A caller with a non-retriable transaction should set keepOpen to false and call pop() anyway, even though the caller does not want a pconn. This forces us to close an available persistent connection, avoiding creating a growing number of open connections when many transactions create (and push) persistent connections but are not retriable and, hence, do not need to pop a connection.
Definition at line 449 of file pconn.cc.
References Comm::Connection::getPeer(), and popStored().
Referenced by HappyConnOpener::reuseOldConnection().
◆ popStored()
|
private |
implements pop() API while disregarding peer standby pools
- Returns
- an open connection or nil
Definition at line 468 of file pconn.cc.
References debugs, IdleConnList::findUseable(), hash_lookup(), hashKeyStr(), key(), notifyManager(), and table.
Referenced by pop().
◆ push()
void PconnPool::push | ( | const Comm::ConnectionPointer & | serverConn, |
const char * | domain | ||
) |
Definition at line 412 of file pconn.cc.
References assert, comm_has_incomplete_write(), conn, debugs, FD_DESC_SZ, fd_note(), fdUsageHigh(), hash_join(), hash_lookup(), hashKeyStr(), key(), LOCAL_ARRAY, notifyManager(), IdleConnList::push(), shutting_down, and table.
Referenced by HttpStateData::processReplyBody(), and PeerPoolMgr::pushNewConnection().
◆ unlinkList()
void PconnPool::unlinkList | ( | IdleConnList * | list | ) |
Definition at line 528 of file pconn.cc.
References assert, IdleConnList::count(), hash_remove_link(), table, and theCount.
Referenced by IdleConnList::~IdleConnList().
Member Data Documentation
◆ descr
|
private |
Definition at line 157 of file pconn.h.
Referenced by ~PconnPool(), and dumpHist().
◆ hist
|
private |
Definition at line 155 of file pconn.h.
Referenced by PconnPool(), dumpHist(), and noteUses().
◆ mgr
|
private |
Definition at line 158 of file pconn.h.
Referenced by notifyManager().
◆ table
|
private |
Definition at line 156 of file pconn.h.
Referenced by PconnPool(), ~PconnPool(), closeN(), dumpHash(), popStored(), push(), and unlinkList().
◆ theCount
|
private |
Definition at line 159 of file pconn.h.
Referenced by count(), noteConnectionAdded(), noteConnectionRemoved(), and unlinkList().
The documentation for this class was generated from the following files: