#include <pconn.h>
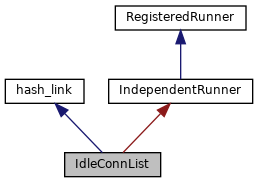
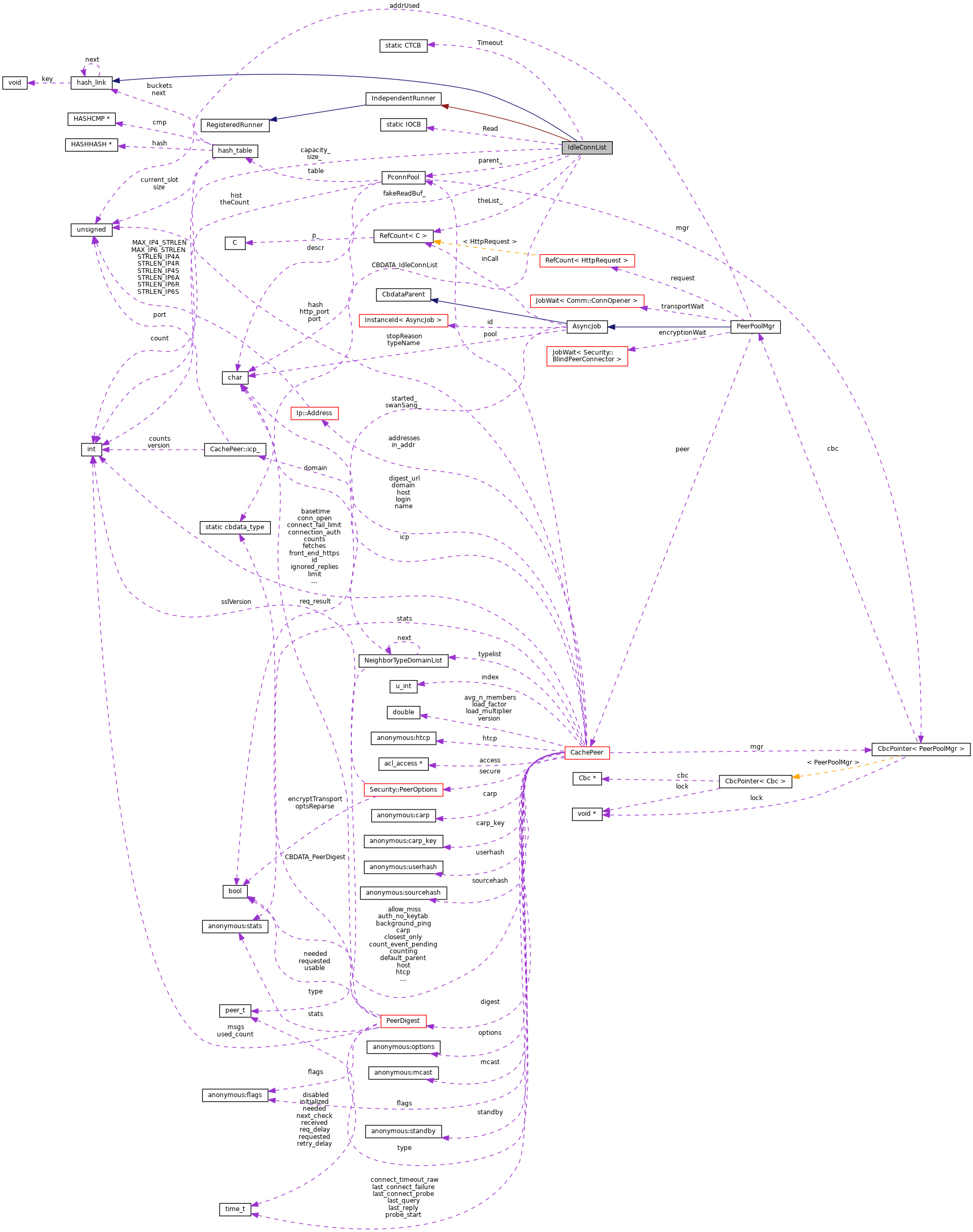
Public Member Functions | |
void * | operator new (size_t size) |
void | operator delete (void *address) |
void * | toCbdata () noexcept |
IdleConnList (const char *key, PconnPool *parent) | |
~IdleConnList () override | |
void | push (const Comm::ConnectionPointer &conn) |
Pass control of the connection to the idle list. More... | |
Comm::ConnectionPointer | pop () |
get first conn which is not pending read fd. More... | |
Comm::ConnectionPointer | findUseable (const Comm::ConnectionPointer &key) |
void | clearHandlers (const Comm::ConnectionPointer &conn) |
int | count () const |
void | closeN (size_t count) |
void | endingShutdown () override |
Public Attributes | |
void * | key |
hash_link * | next |
Private Types | |
typedef void(RegisteredRunner::* | Method) () |
a pointer to one of the above notification methods More... | |
Private Member Functions | |
bool | isAvailable (int i) const |
bool | removeAt (int index) |
int | findIndexOf (const Comm::ConnectionPointer &conn) const |
void | findAndClose (const Comm::ConnectionPointer &conn) |
void | registerRunner () |
void | unregisterRunner () |
unregisters self; safe to call multiple times More... | |
virtual void | bootstrapConfig () |
virtual void | finalizeConfig () |
virtual void | claimMemoryNeeds () |
virtual void | useConfig () |
virtual void | startReconfigure () |
virtual void | syncConfig () |
virtual void | startShutdown () |
virtual void | finishShutdown () |
Meant for cleanup of services needed by the already destroyed objects. More... | |
Private Attributes | |
Comm::ConnectionPointer * | theList_ |
int | capacity_ |
Number of entries theList can currently hold without re-allocating (capacity). More... | |
int | size_ |
PconnPool * | parent_ |
char | fakeReadBuf_ [4096] |
Static Private Attributes | |
static cbdata_type | CBDATA_IdleConnList = CBDATA_UNKNOWN |
static IOCB | Read |
static CTCB | Timeout |
Detailed Description
A list of connections currently open to a particular destination end-point.
Member Typedef Documentation
◆ Method
|
inherited |
Definition at line 94 of file RunnersRegistry.h.
Constructor & Destructor Documentation
◆ IdleConnList()
IdleConnList::IdleConnList | ( | const char * | key, |
PconnPool * | parent | ||
) |
Definition at line 34 of file pconn.cc.
References capacity_, hash_link::key, hash_link::next, IndependentRunner::registerRunner(), theList_, and xstrdup.
◆ ~IdleConnList()
|
override |
Definition at line 50 of file pconn.cc.
References closeN(), hash_link::key, parent_, size_, theList_, PconnPool::unlinkList(), and xfree.
Member Function Documentation
◆ bootstrapConfig()
|
inlinevirtualinherited |
Called right before parsing squid.conf. Meant for initializing/preparing configuration parsing facilities.
Reimplemented in NtlmAuthRr.
Definition at line 46 of file RunnersRegistry.h.
Referenced by SquidMain().
◆ claimMemoryNeeds()
|
inlinevirtualinherited |
Called after finalizeConfig(). Meant for announcing memory reservations before memory is allocated.
Reimplemented in IpcIoRr, and MemStoreRr.
Definition at line 55 of file RunnersRegistry.h.
Referenced by RunConfigUsers().
◆ clearHandlers()
void IdleConnList::clearHandlers | ( | const Comm::ConnectionPointer & | conn | ) |
Definition at line 160 of file pconn.cc.
References comm_read_cancel(), commUnsetConnTimeout(), conn, debugs, and Read.
Referenced by closeN(), findAndClose(), findUseable(), and pop().
◆ closeN()
void IdleConnList::closeN | ( | size_t | count | ) |
Definition at line 113 of file pconn.cc.
References clearHandlers(), conn, debugs, hashKeyStr(), PconnPool::noteConnectionRemoved(), parent_, size_, size_t, and theList_.
Referenced by ~IdleConnList(), and endingShutdown().
◆ count()
|
inline |
◆ endingShutdown()
|
overridevirtual |
Called after shutdown_lifetime grace period ends and before stopping the main loop. At least one main loop iteration is guaranteed after this call. Meant for cleanup and state saving that may require other modules.
Reimplemented from RegisteredRunner.
◆ finalizeConfig()
|
inlinevirtualinherited |
Called after parsing squid.conf. Meant for setting configuration options that depend on other configuration options and were not explicitly configured.
Reimplemented in sslBumpCfgRr, and MemStoreRr.
Definition at line 51 of file RunnersRegistry.h.
Referenced by SquidMain().
◆ findAndClose()
|
private |
Definition at line 284 of file pconn.cc.
References clearHandlers(), conn, findIndexOf(), PconnPool::notifyManager(), parent_, and removeAt().
◆ findIndexOf()
|
private |
Search the list. Matches by FD socket number. Performed from the end of list where newest entries are.
- Return values
-
<0 The connection is not listed >=0 The connection array index
Definition at line 72 of file pconn.cc.
References conn, debugs, Comm::Connection::fd, size_, and theList_.
Referenced by findAndClose().
◆ findUseable()
Comm::ConnectionPointer IdleConnList::findUseable | ( | const Comm::ConnectionPointer & | key | ) |
Search the list for a connection which matches the 'key' details and pop it off the list. The list is created based on remote IP:port hash. This further filters the choices based on specific local-end details requested. If nothing usable is found the a nil pointer is returned.
Definition at line 245 of file pconn.cc.
References assert, clearHandlers(), fd_table, Ip::Address::isAnyAddr(), isAvailable(), Comm::Connection::local, Ip::Address::matchIPAddr(), Ip::Address::port(), removeAt(), size_, and theList_.
Referenced by PconnPool::popStored().
◆ finishShutdown()
|
inlinevirtualinherited |
Definition at line 91 of file RunnersRegistry.h.
Referenced by RunRegistered(), SquidShutdown(), TestRock::tearDown(), and watch_child().
◆ isAvailable()
|
private |
Determine whether an entry in the idle list is available for use. Returns false if the entry is unset, closed or closing.
Definition at line 197 of file pconn.cc.
References COMMIO_FD_READCB, conn, Comm::IsConnOpen(), and theList_.
Referenced by findUseable(), and pop().
◆ operator delete()
◆ operator new()
◆ pop()
Comm::ConnectionPointer IdleConnList::pop | ( | ) |
Definition at line 213 of file pconn.cc.
References clearHandlers(), fd_table, isAvailable(), removeAt(), size_, and theList_.
◆ push()
void IdleConnList::push | ( | const Comm::ConnectionPointer & | conn | ) |
Definition at line 168 of file pconn.cc.
References capacity_, comm_read(), commCbCall(), commSetConnTimeout(), Config, conn, debugs, fakeReadBuf_, PconnPool::noteConnectionAdded(), parent_, Read, SquidConfig::serverIdlePconn, size_, theList_, Timeout, and SquidConfig::Timeout.
Referenced by PconnPool::push().
◆ registerRunner()
|
protectedinherited |
Definition at line 104 of file RunnersRegistry.cc.
References FindRunners(), and RegisterRunner_().
Referenced by ConnStateData::ConnStateData(), IdleConnList(), and Rock::Rebuild::Rebuild().
◆ removeAt()
|
private |
Remove the entry at specified index. May perform a shuffle of list entries to fill the gap.
- Return values
-
false The index is not an in-use entry.
Definition at line 90 of file pconn.cc.
References debugs, hashKeyStr(), PconnPool::noteConnectionRemoved(), parent_, size_, and theList_.
Referenced by findAndClose(), findUseable(), and pop().
◆ startReconfigure()
|
inlinevirtualinherited |
Called after receiving a reconfigure request and before parsing squid.conf. Meant for modules that need to prepare for their configuration being changed [outside their control]. The changes end with the syncConfig() event.
Reimplemented in Dns::ConfigRr.
Definition at line 67 of file RunnersRegistry.h.
Referenced by mainReconfigureStart().
◆ startShutdown()
|
inlinevirtualinherited |
Called after receiving a shutdown request and before stopping the main loop. At least one main loop iteration is guaranteed after this call. Meant for cleanup and state saving that may require other modules.
Reimplemented in ConnStateData, and Rock::Rebuild.
Definition at line 78 of file RunnersRegistry.h.
Referenced by SignalEngine::doShutdown().
◆ syncConfig()
|
inlinevirtualinherited |
Called after parsing squid.conf during reconfiguration. Meant for adjusting the module state based on configuration changes.
Reimplemented in Auth::CredentialCacheRr, and PeerPoolMgrsRr.
Definition at line 71 of file RunnersRegistry.h.
Referenced by mainReconfigureFinish().
◆ toCbdata()
◆ unregisterRunner()
|
protectedinherited |
Definition at line 96 of file RunnersRegistry.cc.
References FindRunners().
Referenced by IndependentRunner::~IndependentRunner().
◆ useConfig()
|
inlinevirtualinherited |
Called after claimMemoryNeeds(). Meant for activating modules and features using a finalized configuration with known memory requirements.
Reimplemented in ClientDbRr, SharedMemPagesRr, Ipc::Mem::RegisteredRunner, MemStoreRr, PeerPoolMgrsRr, SharedSessionCacheRr, and TransientsRr.
Definition at line 60 of file RunnersRegistry.h.
Referenced by RunConfigUsers().
Member Data Documentation
◆ capacity_
|
private |
Number of in-use entries in theList
Definition at line 83 of file pconn.h.
Referenced by IdleConnList(), and push().
◆ CBDATA_IdleConnList
|
staticprivate |
◆ fakeReadBuf_
|
private |
◆ key
|
inherited |
Definition at line 19 of file hash.h.
Referenced by ClientInfo::ClientInfo(), fqdncache_entry::fqdncache_entry(), IdleConnList(), ipcache_entry::ipcache_entry(), net_db_name::net_db_name(), ClientInfo::~ClientInfo(), ExternalACLEntry::~ExternalACLEntry(), fqdncache_entry::~fqdncache_entry(), ~IdleConnList(), net_db_name::~net_db_name(), aclMatchExternal(), Rock::SwapDir::anchorToCache(), MemStore::anchorToCache(), Store::CheckSwapMetaKey(), StoreEntry::clearPublicKeyScope(), Rock::SwapDir::createStoreIO(), CommQuotaQueue::dequeue(), destroyStoreEntry(), CommQuotaQueue::enqueue(), external_acl_cache_add(), StoreEntry::forcePublicKey(), fqdncacheAddEntry(), fqdncacheParse(), StoreEntry::getMD5Text(), hash_join(), hash_remove_link(), StoreEntry::hashDelete(), StoreEntry::hashInsert(), hashKeyStr(), Rock::SwapDir::hasReadableEntry(), htcpQuery(), idnsStartQuery(), ipcacheAddEntry(), ipcacheRelease(), Fs::Ufs::UFSSwapDir::logEntry(), Store::Controller::markedForDeletionAndAbandoned(), my_free(), ipcache_entry::name(), neighborsUdpPing(), netdbHashInsert(), Ipc::StoreMap::openForUpdating(), Store::PackFields(), peerCountMcastPeersCreateAndSend(), StoreEntry::publicKey(), read_passwd_file(), Ipc::StoreMapAnchor::set(), StoreEntry::setPrivateKey(), StoreEntry::setPublicKey(), ClientInfo::setWriteLimiter(), Ident::Start(), MemStore::startCaching(), storeDigestAdd(), storeDigestDel(), storeRebuildParseEntry(), Store::UnpackIndexSwapMeta(), and UFSCleanLog::write().
◆ next
|
inherited |
Definition at line 20 of file hash.h.
Referenced by IdleConnList(), clientdbGC(), Store::LocalSearch::copyBucket(), StoreEntry::dump(), hash_join(), hash_lookup(), hash_next(), hash_remove_link(), and lru_stats().
◆ parent_
|
private |
The pool containing this sub-list. The parent performs all stats accounting, and will delete us when it dies. It persists for the full duration of our existence.
Definition at line 92 of file pconn.h.
Referenced by ~IdleConnList(), closeN(), findAndClose(), push(), and removeAt().
◆ Read
|
staticprivate |
Definition at line 71 of file pconn.h.
Referenced by clearHandlers(), and push().
◆ size_
|
private |
Definition at line 85 of file pconn.h.
Referenced by ~IdleConnList(), closeN(), count(), endingShutdown(), findIndexOf(), findUseable(), pop(), push(), and removeAt().
◆ theList_
|
private |
List of connections we are holding. Sorted as FIFO list for most efficient speeds on pop() and findUsable() The worst-case pop() and scans occur on timeout and link closure events where timing is less critical. Occasional slow additions are okay.
Definition at line 80 of file pconn.h.
Referenced by IdleConnList(), ~IdleConnList(), closeN(), findIndexOf(), findUseable(), isAvailable(), pop(), push(), and removeAt().
◆ Timeout
|
staticprivate |
The documentation for this class was generated from the following files: