#include <Include.h>
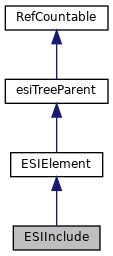
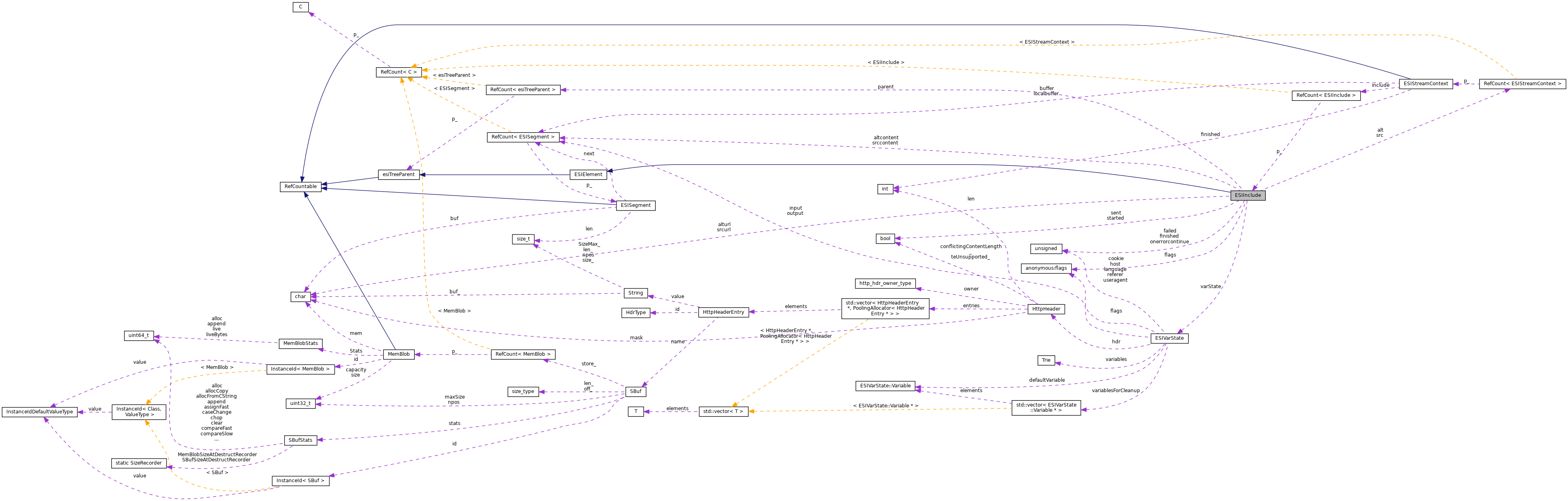
Public Types | |
enum | ESIElementType_t { ESI_ELEMENT_NONE , ESI_ELEMENT_INCLUDE , ESI_ELEMENT_COMMENT , ESI_ELEMENT_REMOVE , ESI_ELEMENT_TRY , ESI_ELEMENT_ATTEMPT , ESI_ELEMENT_EXCEPT , ESI_ELEMENT_VARS , ESI_ELEMENT_CHOOSE , ESI_ELEMENT_WHEN , ESI_ELEMENT_OTHERWISE , ESI_ELEMENT_ASSIGN } |
typedef RefCount< ESIElement > | Pointer |
Public Member Functions | |
ESIInclude (esiTreeParentPtr, int attributes, const char **attr, ESIContext *) | |
~ESIInclude () override | |
void | render (ESISegment::Pointer) override |
esiProcessResult_t | process (int dovars) override |
Pointer | makeCacheable () const override |
Pointer | makeUsable (esiTreeParentPtr, ESIVarState &) const override |
void | subRequestDone (ESIStreamContext::Pointer, bool) |
void | includeFail (ESIStreamContext::Pointer) |
void | finish () override |
virtual bool | addElement (ESIElement::Pointer) |
virtual bool | mayFail () const |
virtual void | provideData (ESISegment::Pointer, ESIElement *) |
virtual void | fail (ESIElement *, char const *=nullptr) |
Static Public Member Functions | |
static ESIElementType_t | IdentifyElement (const char *) |
Public Attributes | |
struct { | |
unsigned int onerrorcontinue:1 | |
unsigned int failed:1 | |
unsigned int finished:1 | |
} | flags |
ESIStreamContext::Pointer | src |
ESIStreamContext::Pointer | alt |
ESISegment::Pointer | srccontent |
ESISegment::Pointer | altcontent |
ESIVarState * | varState |
char * | srcurl |
char * | alturl |
Private Member Functions | |
MEMPROXY_CLASS (ESIInclude) | |
void | Start (ESIStreamContext::Pointer, char const *, ESIVarState *) |
void | start () |
ESIInclude (ESIInclude const &) | |
bool | dataNeeded () const |
void | prepareRequestHeaders (HttpHeader &tempheaders, ESIVarState *vars) |
Private Attributes | |
esiTreeParentPtr | parent |
bool | started |
bool | sent |
Detailed Description
Member Typedef Documentation
◆ Pointer
|
inherited |
Member Enumeration Documentation
◆ ESIElementType_t
|
inherited |
Constructor & Destructor Documentation
◆ ESIInclude() [1/2]
ESIInclude::ESIInclude | ( | esiTreeParentPtr | aParent, |
int | attributes, | ||
const char ** | attr, | ||
ESIContext * | aContext | ||
) |
Definition at line 293 of file Include.cc.
References alt, alturl, assert, cbdataReference, DBG_IMPORTANT, debugs, ESIStreamContextNew(), flags, RefCount< C >::getRaw(), src, srcurl, ESIContext::varState, varState, and xstrdup.
Referenced by makeCacheable(), and makeUsable().
◆ ~ESIInclude()
|
override |
Definition at line 204 of file Include.cc.
References altcontent, alturl, cbdataReferenceDone, debugs, ESISegmentFreeList(), safe_free, srccontent, srcurl, and varState.
◆ ESIInclude() [2/2]
|
private |
Definition at line 243 of file Include.cc.
References alturl, flags, onerrorcontinue, srcurl, and xstrdup.
Member Function Documentation
◆ addElement()
|
inlinevirtualinherited |
Reimplemented in ESIAssign, esiRemove, esiTry, esiChoose, and esiSequence.
Definition at line 64 of file Element.h.
References debugs.
Referenced by ESIContext::addLiteral(), and ESIContext::addStackElement().
◆ dataNeeded()
|
private |
◆ fail()
|
inlinevirtualinherited |
Reimplemented in esiTry, esiChoose, esiSequence, and ESIContext.
Definition at line 33 of file Element.h.
Referenced by esiChoose::fail(), esiSequence::fail(), esiTry::notifyParent(), esiChoose::process(), and subRequestDone().
◆ finish()
|
overridevirtual |
◆ IdentifyElement()
|
staticinherited |
Definition at line 852 of file Esi.cc.
References assert, ESIElement::ESI_ELEMENT_ASSIGN, ESIElement::ESI_ELEMENT_ATTEMPT, ESIElement::ESI_ELEMENT_CHOOSE, ESIElement::ESI_ELEMENT_COMMENT, ESIElement::ESI_ELEMENT_EXCEPT, ESIElement::ESI_ELEMENT_INCLUDE, ESIElement::ESI_ELEMENT_NONE, ESIElement::ESI_ELEMENT_OTHERWISE, ESIElement::ESI_ELEMENT_REMOVE, ESIElement::ESI_ELEMENT_TRY, ESIElement::ESI_ELEMENT_VARS, and ESIElement::ESI_ELEMENT_WHEN.
Referenced by ESIContext::end(), and ESIContext::start().
◆ includeFail()
void ESIInclude::includeFail | ( | ESIStreamContext::Pointer | stream | ) |
Definition at line 423 of file Include.cc.
References subRequestDone().
Referenced by esiBufferRecipient().
◆ makeCacheable()
|
overridevirtual |
◆ makeUsable()
|
overridevirtual |
Implements ESIElement.
Definition at line 227 of file Include.cc.
References ESIInclude(), alt, alturl, cbdataReference, ESIStreamContextNew(), parent, src, srcurl, and varState.
◆ mayFail()
|
inlinevirtualinherited |
Reimplemented in esiSequence.
Definition at line 77 of file Element.h.
Referenced by ESIContext::send().
◆ MEMPROXY_CLASS()
|
private |
◆ prepareRequestHeaders()
|
private |
Definition at line 262 of file Include.cc.
References ESIVarState::header(), HttpHeader::removeHopByHopEntries(), and HttpHeader::update().
Referenced by Start().
◆ process()
|
overridevirtual |
Reimplemented from ESIElement.
Definition at line 398 of file Include.cc.
References debugs, ESI_PROCESS_COMPLETE, ESI_PROCESS_FAILED, ESI_PROCESS_PENDING_MAYFAIL, ESI_PROCESS_PENDING_WONTFAIL, flags, and start().
◆ provideData()
|
inlinevirtualinherited |
Reimplemented in ESIAssign, esiTry, esiChoose, esiSequence, and ESIContext.
Definition at line 28 of file Element.h.
References assert.
Referenced by esiTry::notifyParent(), esiSequence::process(), esiTry::provideData(), esiChoose::provideData(), and subRequestDone().
◆ render()
|
overridevirtual |
Implements ESIElement.
Definition at line 365 of file Include.cc.
References altcontent, assert, debugs, fatal(), flags, RefCount< C >::getRaw(), ESISegment::next, sent, and srccontent.
◆ start()
|
private |
Definition at line 342 of file Include.cc.
References alt, alturl, DBG_IMPORTANT, debugs, flags, RefCount< C >::getRaw(), src, srcurl, Start(), started, and varState.
Referenced by process().
◆ Start()
|
private |
Definition at line 269 of file Include.cc.
References ESISegment::buf, HttpHeader::clean(), clientBeginRequest(), DBG_CRITICAL, debugs, esiBufferDetach, esiBufferRecipient, ESIVarState::extractChar(), ESIVarState::feedData(), RefCount< C >::getRaw(), hoRequest, HTTP_REQBUF_SZ, ESIStreamContext::localbuffer, Http::METHOD_GET, and prepareRequestHeaders().
Referenced by start().
◆ subRequestDone()
void ESIInclude::subRequestDone | ( | ESIStreamContext::Pointer | stream, |
bool | success | ||
) |
Definition at line 435 of file Include.cc.
References alt, altcontent, alturl, assert, dataNeeded(), DBG_CRITICAL, debugs, esiTreeParent::fail(), fatal(), flags, RefCount< C >::getRaw(), ESISegment::ListTransfer(), ESIStreamContext::localbuffer, parent, esiTreeParent::provideData(), sent, src, srccontent, and srcurl.
Referenced by esiBufferRecipient(), and includeFail().
Member Data Documentation
◆ alt
ESIStreamContext::Pointer ESIInclude::alt |
Definition at line 56 of file Include.h.
Referenced by ESIInclude(), makeUsable(), start(), and subRequestDone().
◆ altcontent
ESISegment::Pointer ESIInclude::altcontent |
Definition at line 58 of file Include.h.
Referenced by ~ESIInclude(), render(), and subRequestDone().
◆ alturl
char * ESIInclude::alturl |
Definition at line 60 of file Include.h.
Referenced by ESIInclude(), ~ESIInclude(), makeUsable(), start(), and subRequestDone().
◆ failed
◆ finished
◆
struct { ... } ESIInclude::flags |
Referenced by ESIInclude(), dataNeeded(), process(), render(), start(), and subRequestDone().
◆ onerrorcontinue
unsigned int ESIInclude::onerrorcontinue |
Definition at line 51 of file Include.h.
Referenced by ESIInclude().
◆ parent
|
private |
Definition at line 66 of file Include.h.
Referenced by finish(), makeUsable(), and subRequestDone().
◆ sent
|
private |
Definition at line 69 of file Include.h.
Referenced by render(), and subRequestDone().
◆ src
ESIStreamContext::Pointer ESIInclude::src |
Definition at line 55 of file Include.h.
Referenced by ESIInclude(), makeUsable(), start(), and subRequestDone().
◆ srccontent
ESISegment::Pointer ESIInclude::srccontent |
Definition at line 57 of file Include.h.
Referenced by ~ESIInclude(), render(), and subRequestDone().
◆ srcurl
char* ESIInclude::srcurl |
Definition at line 60 of file Include.h.
Referenced by ESIInclude(), ~ESIInclude(), makeUsable(), start(), and subRequestDone().
◆ started
◆ varState
ESIVarState* ESIInclude::varState |
Definition at line 59 of file Include.h.
Referenced by ESIInclude(), ~ESIInclude(), makeUsable(), and start().
The documentation for this class was generated from the following files:
- src/esi/Include.h
- src/esi/Include.cc