#include <VarState.h>
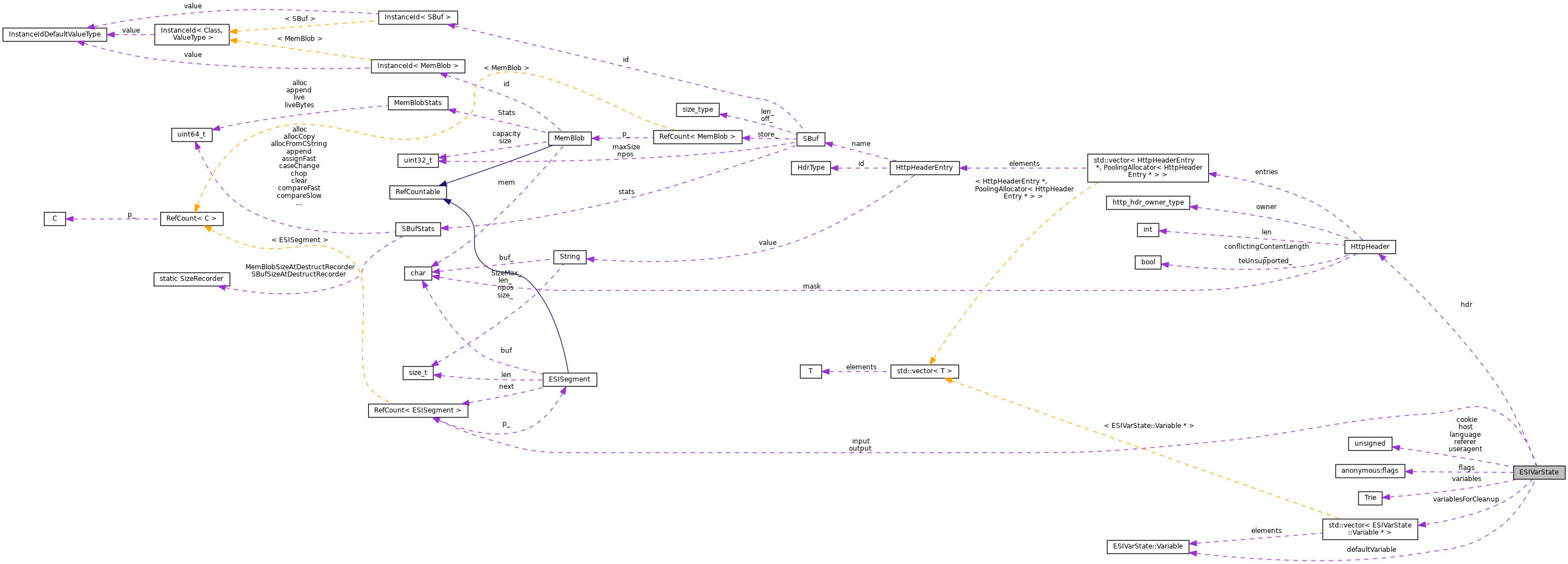
Classes | |
class | Variable |
Public Member Functions | |
ESIVarState (HttpHeader const *hdr, char const *uri) | |
~ESIVarState () | |
ESISegment::Pointer | extractList () |
char * | extractChar () |
void | feedData (const char *buf, size_t len) |
void | buildVary (HttpReply *rep) |
void | addVariable (char const *, size_t, Variable *) |
void | removeVariable (String const &) |
void | cookieUsed () |
void | hostUsed () |
void | languageUsed () |
void | refererUsed () |
void | useragentUsed () |
ESISegment::Pointer & | getOutput () |
HttpHeader & | header () |
Variable * | GetVar (char const *s, int len) |
Private Member Functions | |
CBDATA_CLASS (ESIVarState) | |
void | doIt () |
void | setupUserAgent () |
Private Attributes | |
ESISegment::Pointer | input |
ESISegment::Pointer | output |
HttpHeader | hdr |
struct { | |
unsigned int language:1 | |
unsigned int cookie:1 | |
unsigned int host:1 | |
unsigned int referer:1 | |
unsigned int useragent:1 | |
} | flags |
Trie | variables |
std::vector< Variable * > | variablesForCleanup |
Variable * | defaultVariable |
Detailed Description
Definition at line 34 of file VarState.h.
Constructor & Destructor Documentation
◆ ESIVarState()
ESIVarState::ESIVarState | ( | HttpHeader const * | hdr, |
char const * | uri | ||
) |
Definition at line 242 of file VarState.cc.
References addVariable(), HttpHeader::append(), defaultVariable, flags, and hdr.
◆ ~ESIVarState()
ESIVarState::~ESIVarState | ( | ) |
Definition at line 133 of file VarState.cc.
References HttpHeader::clean(), defaultVariable, ESISegmentFreeList(), hdr, input, output, and variablesForCleanup.
Member Function Documentation
◆ addVariable()
Definition at line 282 of file VarState.cc.
References String::assign(), removeVariable(), variables, and variablesForCleanup.
Referenced by ESIVarState(), and ESIAssign::process().
◆ buildVary()
void ESIVarState::buildVary | ( | HttpReply * | rep | ) |
Definition at line 810 of file VarState.cc.
References flags, HttpHeader::getList(), Http::Message::header, HttpHeader::putStr(), String::size(), and Http::VARY.
Referenced by ESIContext::send().
◆ CBDATA_CLASS()
|
private |
◆ cookieUsed()
void ESIVarState::cookieUsed | ( | ) |
Definition at line 46 of file VarState.cc.
References flags.
Referenced by ESIVariableCookie::eval().
◆ doIt()
|
private |
Definition at line 598 of file VarState.cc.
References ESIVariableProcessor::doIt(), ESISegmentFreeList(), input, ESISegment::listToChar(), output, safe_free, and variables.
Referenced by extractChar(), and extractList().
◆ extractChar()
char * ESIVarState::extractChar | ( | ) |
Definition at line 117 of file VarState.cc.
References debugs, doIt(), ESISegmentFreeList(), fatal(), RefCount< C >::getRaw(), input, ESISegment::listToChar(), and output.
Referenced by esiWhen::evaluate(), ESIAssign::evaluateVariable(), and ESIInclude::Start().
◆ extractList()
ESISegment::Pointer ESIVarState::extractList | ( | ) |
Definition at line 107 of file VarState.cc.
References debugs, doIt(), and output.
Referenced by esiLiteral::process().
◆ feedData()
void ESIVarState::feedData | ( | const char * | buf, |
size_t | len | ||
) |
Definition at line 99 of file VarState.cc.
References debugs, input, and ESISegment::ListAppend().
Referenced by esiWhen::evaluate(), ESIAssign::evaluateVariable(), esiLiteral::process(), and ESIInclude::Start().
◆ getOutput()
ESISegment::Pointer & ESIVarState::getOutput | ( | ) |
Definition at line 76 of file VarState.cc.
References output.
Referenced by ESIVarState::Variable::eval(), ESIVariableExpression::eval(), ESIVariableCookie::eval(), ESIVariableHost::eval(), ESIVariableLanguage::eval(), ESIVariableQuery::eval(), ESIVariableReferer::eval(), and ESIVariableUserAgent::eval().
◆ GetVar()
ESIVarState::Variable * ESIVarState::GetVar | ( | char const * | s, |
int | len | ||
) |
Definition at line 585 of file VarState.cc.
References assert, defaultVariable, and variables.
Referenced by ESIVariableProcessor::ESIVariableProcessor(), and ESIVariableProcessor::doFunction().
◆ header()
HttpHeader & ESIVarState::header | ( | ) |
Definition at line 70 of file VarState.cc.
References hdr.
Referenced by ESIVariableUserAgent::ESIVariableUserAgent(), ESIVariableCookie::eval(), ESIVariableHost::eval(), ESIVariableLanguage::eval(), ESIVariableReferer::eval(), ESIVariableUserAgent::eval(), and ESIInclude::prepareRequestHeaders().
◆ hostUsed()
void ESIVarState::hostUsed | ( | ) |
◆ languageUsed()
void ESIVarState::languageUsed | ( | ) |
Definition at line 52 of file VarState.cc.
References flags.
Referenced by ESIVariableLanguage::eval().
◆ refererUsed()
void ESIVarState::refererUsed | ( | ) |
Definition at line 58 of file VarState.cc.
References flags.
Referenced by ESIVariableReferer::eval().
◆ removeVariable()
void ESIVarState::removeVariable | ( | String const & | name | ) |
Definition at line 267 of file VarState.cc.
References String::rawBuf(), String::size(), and variables.
Referenced by addVariable().
◆ setupUserAgent()
|
private |
◆ useragentUsed()
void ESIVarState::useragentUsed | ( | ) |
Definition at line 64 of file VarState.cc.
References flags.
Referenced by ESIVariableUserAgent::eval().
Member Data Documentation
◆ cookie
unsigned int ESIVarState::cookie |
Definition at line 67 of file VarState.h.
◆ defaultVariable
|
private |
Definition at line 97 of file VarState.h.
Referenced by ESIVarState(), ~ESIVarState(), and GetVar().
◆
struct { ... } ESIVarState::flags |
Referenced by ESIVarState(), buildVary(), cookieUsed(), hostUsed(), languageUsed(), refererUsed(), and useragentUsed().
◆ hdr
|
private |
Definition at line 63 of file VarState.h.
Referenced by ESIVarState(), ~ESIVarState(), and header().
◆ host
unsigned int ESIVarState::host |
Definition at line 68 of file VarState.h.
◆ input
|
private |
Definition at line 61 of file VarState.h.
Referenced by ~ESIVarState(), doIt(), extractChar(), and feedData().
◆ language
unsigned int ESIVarState::language |
Definition at line 66 of file VarState.h.
◆ output
|
private |
Definition at line 62 of file VarState.h.
Referenced by ~ESIVarState(), doIt(), extractChar(), extractList(), and getOutput().
◆ referer
unsigned int ESIVarState::referer |
Definition at line 69 of file VarState.h.
◆ useragent
unsigned int ESIVarState::useragent |
Definition at line 70 of file VarState.h.
◆ variables
|
private |
Definition at line 95 of file VarState.h.
Referenced by addVariable(), doIt(), GetVar(), and removeVariable().
◆ variablesForCleanup
|
private |
Definition at line 96 of file VarState.h.
Referenced by ~ESIVarState(), and addVariable().
The documentation for this class was generated from the following files:
- src/esi/VarState.h
- src/esi/VarState.cc