#include <Segment.h>
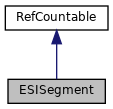
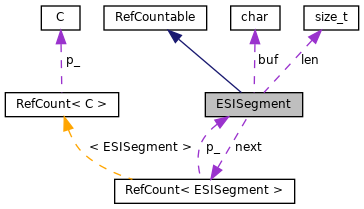
Public Types | |
typedef RefCount< ESISegment > | Pointer |
Public Member Functions | |
ESISegment () | |
ESISegment (ESISegment const &) | |
~ESISegment () override | |
ESISegment::Pointer | cloneList () const |
char * | listToChar () const |
void | listAppend (char const *s, size_t length) |
void | adsorbList (ESISegment::Pointer from) |
size_t | space () const |
size_t | append (char const *, size_t) |
size_t | append (Pointer) |
ESISegment const * | tail () const |
ESISegment * | tail () |
void | dumpToLog () const |
Static Public Member Functions | |
static void | ListAppend (Pointer &, char const *, size_t) |
static void | ListTransfer (Pointer &from, Pointer &to) |
Public Attributes | |
char | buf [HTTP_REQBUF_SZ] |
size_t | len |
Pointer | next |
Private Member Functions | |
CBDATA_CLASS (ESISegment) | |
size_t | listLength () const |
void | dumpOne () const |
Detailed Description
Member Typedef Documentation
◆ Pointer
typedef RefCount<ESISegment> ESISegment::Pointer |
Constructor & Destructor Documentation
◆ ESISegment() [1/2]
|
inline |
Definition at line 30 of file Segment.h.
References buf.
Referenced by cloneList(), listAppend(), and ListAppend().
◆ ESISegment() [2/2]
ESISegment::ESISegment | ( | ESISegment const & | old | ) |
Definition at line 174 of file Segment.cc.
◆ ~ESISegment()
Member Function Documentation
◆ adsorbList()
void ESISegment::adsorbList | ( | ESISegment::Pointer | from | ) |
Definition at line 36 of file Segment.cc.
References append(), assert, RefCount< C >::getRaw(), len, next, and space().
Referenced by ListTransfer().
◆ append() [1/2]
Definition at line 138 of file Segment.cc.
References buf, len, min(), and space().
Referenced by esiLiteral::esiLiteral(), ESISegment(), adsorbList(), append(), ESIContext::fail(), and listAppend().
◆ append() [2/2]
size_t ESISegment::append | ( | ESISegment::Pointer | from | ) |
Definition at line 147 of file Segment.cc.
◆ CBDATA_CLASS()
|
private |
◆ cloneList()
ESISegment::Pointer ESISegment::cloneList | ( | ) | const |
Definition at line 130 of file Segment.cc.
References ESISegment(), cloneList(), RefCount< C >::getRaw(), and next.
Referenced by cloneList().
◆ dumpOne()
|
private |
Definition at line 191 of file Segment.cc.
References String::assign(), buf, debugs, and len.
Referenced by dumpToLog().
◆ dumpToLog()
void ESISegment::dumpToLog | ( | ) | const |
Definition at line 180 of file Segment.cc.
References dumpOne(), RefCount< C >::getRaw(), and next.
◆ listAppend()
void ESISegment::listAppend | ( | char const * | s, |
size_t | length | ||
) |
Definition at line 101 of file Segment.cc.
References ESISegment(), append(), assert, RefCount< C >::getRaw(), next, and space().
◆ ListAppend()
|
static |
Definition at line 120 of file Segment.cc.
References ESISegment(), head, and len.
Referenced by ESIVariableProcessor::doIt(), ESIVarState::Variable::eval(), ESIVariableExpression::eval(), ESIVariableCookie::eval(), ESIVariableHost::eval(), ESIVariableLanguage::eval(), ESIVariableQuery::eval(), ESIVariableReferer::eval(), ESIVariableUserAgent::eval(), and ESIVarState::feedData().
◆ listLength()
|
private |
Definition at line 67 of file Segment.cc.
References RefCount< C >::getRaw(), len, and next.
Referenced by listToChar().
◆ listToChar()
char * ESISegment::listToChar | ( | ) | const |
Definition at line 81 of file Segment.cc.
References assert, buf, RefCount< C >::getRaw(), len, listLength(), next, and xmalloc.
Referenced by ESIVarState::doIt(), ESIVarState::extractChar(), and ESIAssign::provideData().
◆ ListTransfer()
|
static |
Definition at line 53 of file Segment.cc.
References adsorbList(), RefCount< C >::getRaw(), and tail().
Referenced by esiSequence::addElement(), esiTry::provideData(), esiTry::render(), and ESIInclude::subRequestDone().
◆ space()
size_t ESISegment::space | ( | ) | const |
Definition at line 29 of file Segment.cc.
References assert, buf, and len.
Referenced by adsorbList(), append(), and listAppend().
◆ tail() [1/2]
ESISegment * ESISegment::tail | ( | ) |
Definition at line 164 of file Segment.cc.
References RefCount< C >::getRaw(), and next.
◆ tail() [2/2]
ESISegment const * ESISegment::tail | ( | ) | const |
Definition at line 153 of file Segment.cc.
References RefCount< C >::getRaw(), and next.
Referenced by esiBufferRecipient(), ESIContext::fixupOutboundTail(), ListTransfer(), and esiSequence::render().
Member Data Documentation
◆ buf
char ESISegment::buf[HTTP_REQBUF_SZ] |
Definition at line 40 of file Segment.h.
Referenced by esiLiteral::esiLiteral(), ESISegment(), append(), dumpOne(), esiBufferRecipient(), esiProcessStream(), esiStreamRead(), listToChar(), ESIContext::parseOneBuffer(), esiLiteral::process(), ESIContext::send(), space(), and ESIInclude::Start().
◆ len
size_t ESISegment::len |
Definition at line 41 of file Segment.h.
Referenced by esiLiteral::esiLiteral(), ESISegment(), adsorbList(), append(), dumpOne(), esiBufferRecipient(), esiProcessStream(), esiStreamRead(), esiStreamStatus(), ListAppend(), listLength(), listToChar(), ESIContext::parseOneBuffer(), esiLiteral::process(), esiSequence::process(), ESIContext::send(), space(), and ESIContext::trimBlanks().
◆ next
Pointer ESISegment::next |
Definition at line 42 of file Segment.h.
Referenced by esiLiteral::esiLiteral(), adsorbList(), ESIContext::appendOutboundData(), cloneList(), dumpToLog(), esiBufferRecipient(), esiProcessStream(), ESISegmentFreeList(), listAppend(), listLength(), listToChar(), ESIContext::parseOneBuffer(), esiLiteral::process(), esiSequence::process(), esiChoose::render(), ESIInclude::render(), esiLiteral::render(), esiSequence::render(), ESIContext::send(), tail(), and ESIContext::trimBlanks().
The documentation for this class was generated from the following files:
- src/esi/Segment.h
- src/esi/Segment.cc